c++ 读取加密文件
时间: 2024-10-19 08:10:49 浏览: 26
在 C++ 中,读取加密文件通常涉及到两个步骤:首先解密数据,然后读取解密后的内容。如果你有一个预定义的加密算法(比如 AES、RSA 等),你需要使用相应的库(如 OpenSSL 或者 Crypto++)来进行解密操作。
以下是一个基本示例,假设你已经获取了加密的数据和对应的密钥:
```cpp
#include <iostream>
#include <fstream>
#include <openssl/aes.h> // 使用 OpenSSL 进行 AES 解密
// 使用 OpenSSL 的 AES 加密函数 (这里仅作演示)
std::string encrypt(const std::string& plaintext, const std::string& key) {
// ... 实现 AES 加密逻辑 ...
}
// 使用 OpenSSL 的 AES 解密函数 (这里仅作演示)
std::string decrypt(const std::string& ciphertext, const std::string& key) {
AES_KEY aes_key;
AES_set_encrypt_key((const unsigned char*)key.c_str(), 128, &aes_key); // 128位AES
size_t decrypted_length = strlen(ciphertext);
unsigned char output[decrypted_length];
AES_decrypt(reinterpret_cast<const unsigned char*>(ciphertext.c_str()), output, &aes_key);
return std::string(output, decrypted_length);
}
int main() {
try {
std::ifstream encrypted_file("encrypted_file.txt", std::ios::binary);
if (!encrypted_file.is_open()) {
std::cerr << "Failed to open file." << std::endl;
return 1;
}
std::string encryption_key = "your_secret_key";
std::string decrypted_content = decrypt(encrypted_file.readsome(), encryption_key); // 替换readsome()为实际读取的加密数据
std::ofstream decrypted_file("decrypted_content.txt", std::ios::binary);
decrypted_file.write(decrypted_content.c_str(), decrypted_content.size());
encrypted_file.close();
decrypted_file.close();
std::cout << "Decryption successful. Decrypted content saved to 'decrypted_content.txt'" << std::endl;
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
阅读全文
相关推荐
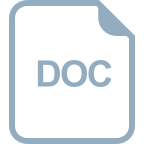
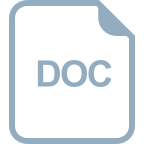
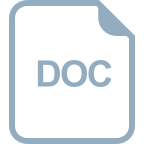

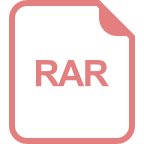
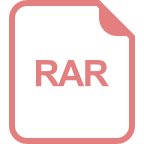
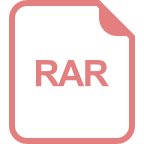
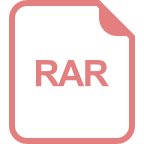
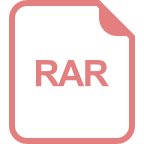
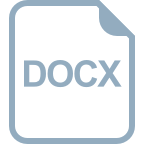
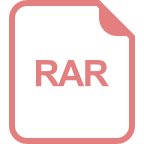
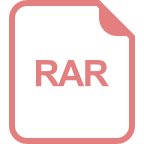
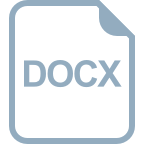



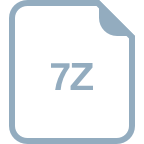
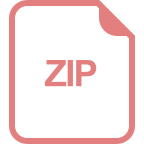
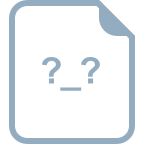