c语言编写个人所得税代码
时间: 2024-01-19 11:02:31 浏览: 79
以下是c语言编写个人所得税代码的范例:
```c
#include <stdio.h>
int main() {
double income, tax;
printf("请输入您的收入\n");
scanf("%lf", &income);
if (income < 1000) {
tax = 0;
} else if (income >= 1000 && income < 2000) {
tax = (income - 1000) * 0.05;
} else if (income >= 2000 && income < 4000) {
tax = (income - 2000) * 0.1 + 50;
} else if (income >= 4000 && income < 6000) {
tax = (income - 4000) * 0.15 + 250;
} else {
tax = (income - 6000) * 0.2 + 550;
}
income -= tax;
if (tax == 0) {
printf("您无需交税,实际收入为%.2f元", income);
} else {
printf("您应纳的税额为%.2f,实际收入为%.2f", tax, income);
}
return 0;
}
```
相关问题
写一个C语言计算个人所得税的代码
编写一个完整的C语言程序来计算个人所得税可能会比较复杂,因为涉及到多种情况和数据结构。这里是一个简化的例子,仅展示如何处理月度工资所得税的基本逻辑,实际应用中需要更详细的输入验证和税率查找机制。
```c
#include <stdio.h>
// 假设税率表和速算扣除数固定
const double rate[] = {0, 3, 10, 20, 25, 30, 35};
const double deduction[] = {0, 210, 1410, 3192, 6132, 12792, 45480};
double calculate_tax(double income) {
// 超过一定数额后税率开始上升
int tax_bracket = (int)(income / 5000);
// 计算税额
double tax = income * rate[tax_bracket] / 100 - deduction[tax_bracket];
return tax;
}
int main() {
double monthly_income;
printf("请输入月收入(单位:元):");
scanf("%lf", &monthly_income);
if (monthly_income <= 5000) {
printf("本月无需缴纳个人所得税。\n");
} else {
double tax_amount = calculate_tax(monthly_income);
printf("本月应缴个人所得税为%.2f元。\n", tax_amount);
}
return 0;
}
```
用 C语言编写一个测试个人所得税计算程序,
可以使用以下代码:
#include <stdio.h>
int main() {
float income, tax;
printf("请输入您的年收入:");
scanf("%f", &income);
if (income <= 0) {
printf("您输入的收入不合法!\n");
return 0;
}
if (income <= 36000) {
tax = income * 0.03;
} else if (income <= 144000) {
tax = 1080 + (income - 36000) * 0.1;
} else if (income <= 300000) {
tax = 9900 + (income - 144000) * 0.2;
} else if (income <= 420000) {
tax = 27900 + (income - 300000) * 0.25;
} else if (income <= 660000) {
tax = 47900 + (income - 420000) * 0.3;
} else if (income <= 960000) {
tax = 85900 + (income - 660000) * 0.35;
} else {
tax = 181900 + (income - 960000) * 0.45;
}
printf("您的个人所得税为:%.2f 元\n", tax);
return 0;
}
这个程序可以根据您输入的年收入计算出您需要缴纳的个人所得税。根据中国税法规定,个人所得税的税率是分档计算的,不同的收入段有不同的税率。
阅读全文
相关推荐



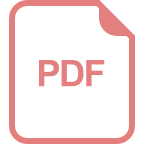
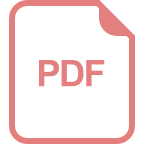
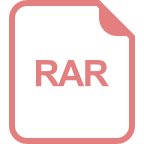
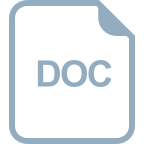
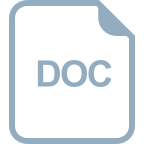
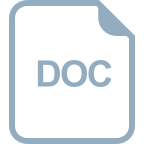



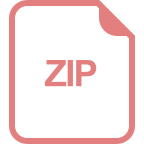
