c语言写一个根据字符串1-44查表返回对应字符串的函数
时间: 2024-10-25 19:13:09 浏览: 28
在C语言中,你可以创建一个数组来存储字符串对应的数值,然后编写一个函数来接收输入字符串并在数组中查找匹配项。这里是一个简单的例子:
```c
#include <stdio.h>
#include <string.h>
#define TABLE_SIZE 45
char* string_table[TABLE_SIZE] = {
"Invalid", "One", "Two", "Three", ..., "FortyFour" // 填充完整字符串
};
// 函数声明
char* get_string_from_number(const char* input);
int main() {
char input[5]; // 输入最多4位数字,加一个终止符
printf("Enter a number from 1 to 44: ");
fgets(input, sizeof(input), stdin);
input[strcspn(input, "\n")] = '\0'; // 清除换行符
char* result = get_string_from_number(input);
if (result) {
printf("The corresponding string is: %s\n", result);
} else {
printf("Invalid input!\n");
}
return 0;
}
// 查找并返回对应的字符串
char* get_string_from_number(const char* input) {
int num = atoi(input); // 将输入转换为整数
if (num >= 1 && num <= 44) { // 验证输入是否在范围内
return string_table[num - 1];
} else {
return NULL; // 如果不在范围,则返回NULL表示无效
}
}
```
在这个例子中,`get_string_from_number`函数会尝试将用户输入的字符串转换成整数,并从预定义的字符串数组中找到相应的字符串。如果输入超出范围,函数会返回`NULL`。
阅读全文
相关推荐















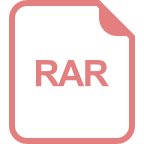

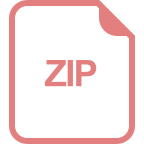
