用JSP编写一个实验页面计数的计数器,要求当刷新页面时,不增加计数。不使用get Attribute方法
时间: 2024-10-10 10:16:49 浏览: 37
在JSP中创建一个不使用`getAttribute`方法的简单计数器,可以利用Session存储数据来实现。下面是一个基本的步骤:
1. 首先,在服务器端(Java),我们需要一个Servlet来处理请求并更新计数:
```java
import javax.servlet.*;
import java.io.IOException;
@WebServlet("/counter")
public class CounterServlet extends HttpServlet {
private int count = 0; // 初始化计数
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
++count;
HttpSession session = request.getSession();
session.setAttribute("counter", count); // 将计数保存到Session
response.sendRedirect(request.getContextPath() + "/counter.jsp"); // 重定向回JSP页面
}
}
```
2. 创建一个名为`counter.jsp`的JSP页面,用于显示计数并处理GET请求:
```jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<!DOCTYPE html>
<html>
<head>
<title>计数器</title>
</head>
<body>
<h1>点击次数: <%= (Integer)session.getAttribute("counter") %></h1>
<a href="<c:url value="/counter" />">刷新页面</a>
</body>
</html>
```
注意这里使用了JSTL标签 `<c:url>` 来避免直接暴露Servlet URL。
**相关问题:**
1. JSP如何通过Session保持用户状态?
2. JSP和Servlet协作时,为什么通常使用POST而不是GET?
3. 在这个例子中,如果需要在多个页面之间共享计数器,应该如何改进?
阅读全文
相关推荐
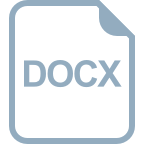
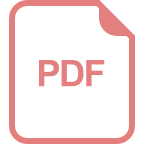
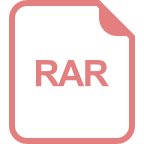
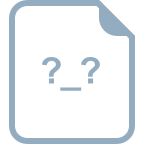
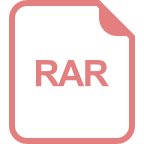
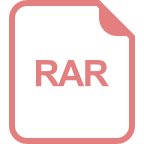
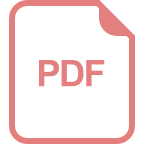
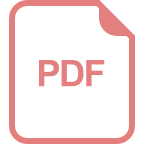
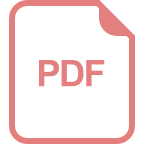
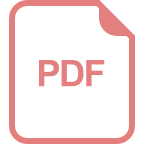
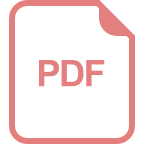
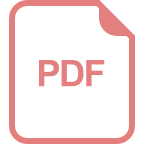
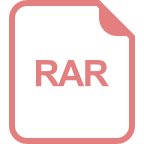
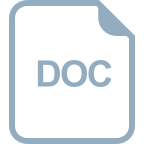
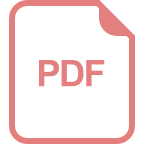


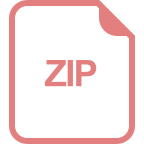