在二维数组中输入三个任意长度的字符串用c语言完成
时间: 2024-10-02 16:03:36 浏览: 45
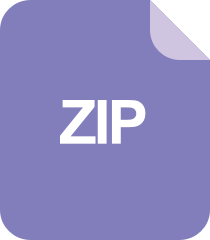
c语言基础-c语言编程基础之二维字符串数组示例-Bigtram分词.zip
在C语言中,处理任意长度的二维字符数组并输入三个字符串可以使用动态内存分配和循环结构来实现。下面是一个简单的示例,展示了如何完成这个任务:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_ROWS 10
#define MAX_COLS 50
void input_strings(char** arr, int rows, int cols) {
for (int i = 0; i < rows; ++i) {
char* temp = malloc(cols * sizeof(char)); // 动态分配行内字符串空间
if (!temp) {
printf("Memory allocation failed.\n");
return;
}
scanf("%s", temp); // 输入一行字符串
arr[i] = temp;
if (strlen(arr[i]) >= cols) { // 检查是否超过指定列数
free(temp);
continue;
}
// 如果字符串长度小于列数,则填充空格
while (strlen(arr[i]) < cols) {
arr[i][strlen(arr[i])] = ' '; // 添加空格
}
arr[i][cols] = '\0'; // 结束字符串
}
}
int main() {
int rows, cols;
printf("Enter the number of rows and columns (separated by space): ");
scanf("%d %d", &rows, &cols);
if (rows > MAX_ROWS || cols > MAX_COLS) {
printf("Row or column count exceeds the limit.\n");
return 1;
}
char** two_dim_array = malloc(rows * sizeof(char*)); // 动态分配二维数组
if (!two_dim_array) {
printf("Memory allocation failed.\n");
return 1;
}
input_strings(two_dim_array, rows, cols);
printf("\nInputted strings:\n");
for (int i = 0; i < rows; ++i) {
printf("%s\n", two_dim_array[i]);
}
// 清理内存
for (int i = 0; i < rows; ++i) {
free(two_dim_array[i]);
}
free(two_dim_array);
return 0;
}
阅读全文
相关推荐
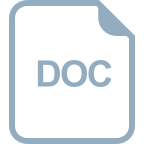
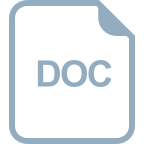
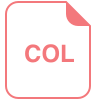

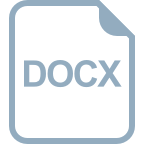
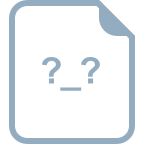
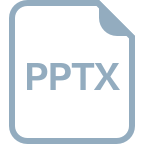
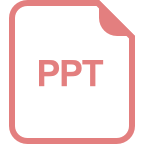
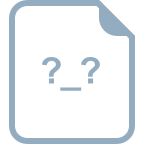
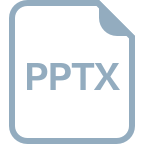
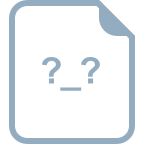
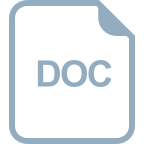
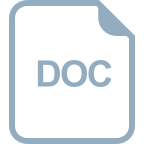
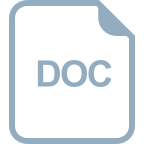
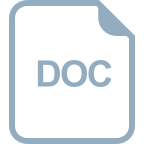
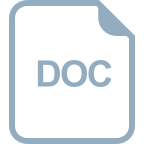
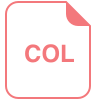
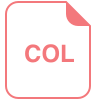