pyside6 treewidget 與qtsql聯動,請實例化,並注釋
时间: 2024-09-08 14:00:18 浏览: 19
在PySide6中,您可以使用`TreeWidget`组件来创建树状结构的视图,并与`QtSql`模块联动来处理数据库操作。以下是一个简单的示例,展示了如何创建一个`TreeWidget`,并将数据库中的数据填充到这个视图中。
首先,确保您的环境中安装了PySide6和QtSql模块。然后,您需要创建一个数据库连接,并创建一个简单的数据库表。这里为了简单起见,我们使用SQLite数据库。
示例代码如下:
```python
import sys
from PySide6.QtWidgets import QApplication, QMainWindow, QHeaderView, QTreeView, QTableWidget, QVBoxLayout, QWidget
from PySide6.QtSql import QSqlDatabase, QSqlTableModel
class TreeWidgetExample(QMainWindow):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
# 创建数据库连接
db = QSqlDatabase.addDatabase('QSQLITE')
db.setDatabaseName('example.db')
if not db.open():
print("无法打开数据库")
sys.exit(1)
# 创建数据库模型
model = QSqlTableModel(self)
model.setTable('items') # 假设我们有一个名为items的表
model.select()
# 创建树形视图
treeView = QTreeView(self)
treeView.setModel(model)
# 由于数据库模型可能不支持树形视图的层级结构,这里我们用QTableWidget代替
tableWidget = QTableWidget()
tableWidget.setHorizontalHeaderLabels(['ID', 'Name', 'Description'])
tableWidget.setColumnWidth(0, 100)
tableWidget.setColumnWidth(1, 200)
# 将数据填充到tableWidget
for row in range(model.rowCount()):
item_id = model.index(row, 0).data()
item_name = model.index(row, 1).data()
item_desc = model.index(row, 2).data()
tableWidget.insertRow(row)
tableWidget.setItem(row, 0, self.tableWidgetItem(item_id))
tableWidget.setItem(row, 1, self.tableWidgetItem(item_name))
tableWidget.setItem(row, 2, self.tableWidgetItem(item_desc))
# 设置树形视图的头视图为可显示表头
treeView.setHeaderHidden(False)
# 设置布局
layout = QVBoxLayout()
layout.addWidget(tableWidget)
container = QWidget()
container.setLayout(layout)
self.setCentralWidget(container)
def tableWidgetItem(self, text):
"""创建表格项"""
item = tableWidget.item(row, column)
if item is None:
item = tableWidget.dequeueReusableCell()
item.setText(text)
return item
def main():
app = QApplication(sys.argv)
ex = TreeWidgetExample()
ex.show()
sys.exit(app.exec())
if __name__ == '__main__':
main()
```
注意:在这个例子中,我们使用了`QTableWidget`代替`QTreeView`,因为`QSqlTableModel`并不直接支持层级结构数据。如果您的数据本身就是层级结构的,您可能需要使用`QSqlRelationalTableModel`或自定义模型来实现树形结构。
请根据您的实际数据库表结构调整表名和字段名。
相关推荐
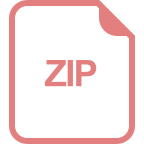
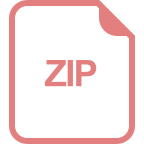














