最短哈密顿回路算法C语言实现
时间: 2025-01-04 13:37:41 浏览: 6
最短哈密顿回路是一种图论问题,目标是在给定的无向加权图中找到一条经过所有顶点恰好一次并返回起点的路径,使得总权重最小。在C语言中实现这样一个算法通常是结合搜索策略(如深度优先搜索、广度优先搜索)和贪心策略,比如Prim算法或Kruskal算法。
Prim算法可以从任意一个顶点开始,每次添加到最小边的一端,直到形成一个包含所有顶点的连通分量。这个过程可以转化为一个优先队列操作,例如用堆数据结构来存储未加入的边及其终点。
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct {
int u, v; // 边的两端
int weight;
} Edge;
typedef struct Node {
int id;
Edge* edges;
int nEdges;
int minDistance; // 这个节点到达已连接部分的最短距离
bool visited;
} Node;
// ...其他辅助函数...
void prim(Node* graph, int numVertices) {
// 初始化每个顶点的距离为无穷大,只有一个起始点例外
for (int i = 0; i < numVertices; ++i) {
graph[i].minDistance = INT_MAX;
}
graph[0].minDistance = 0;
// 创建优先队列,并将起始点入队
Edge* minEdge = NULL;
priority_queue<Edge*, vector<Edge*>, compareEdges> pq;
for (int i = 0; i < numVertices; ++i) {
if (graph[i].minDistance != INT_MAX) {
pq.push(&graph[i].edges[0]);
}
}
while (!pq.empty()) {
Edge* currentEdge = pq.top(); pq.pop();
int u = currentEdge->u;
int v = currentEdge->v;
int distance = currentEdge->weight;
if (distance < graph[u].minDistance) {
graph[u].minDistance = distance;
graph[v].visited = true; // 更新邻接顶点的访问状态
// 如果邻接顶点还没添加到树中,更新其距离并入队
if (!graph[v].visited) {
graph[v].minDistance = distance + graph[u].minDistance;
pq.push(&graph[v].edges[0]);
}
}
}
}
// ...更多相关函数和主函数...
阅读全文
相关推荐
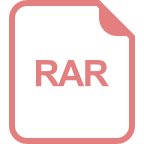










