用遗传算法解决背包问题
时间: 2023-12-16 12:05:43 浏览: 108
遗传算法是一种基于自然选择和遗传学原理的优化算法,可以用于解决背包问题。具体步骤如下:
1. 初始化种群:随机生成一定数量的个体,每个个体代表一种背包的组合方案。
2. 适应度函数:计算每个个体的适应度,即背包中物品的总价值。
3. 选择操作:根据适应度函数的值,选择一定数量的个体作为下一代的父代。
4. 交叉操作:对父代进行交叉操作,生成新的个体。
5. 变异操作:对新的个体进行变异操作,引入新的基因。
6. 重复步骤2-5,直到达到预设的终止条件。
7. 输出最优解:输出适应度函数值最大的个体,即为最优解。
下面是一个使用遗传算法解决背包问题的Python代码示例:
```python
import random
# 背包容量
capacity = 80
# 物品列表,每个元素为元组,第一个元素为物品重量,第二个元素为物品价值
items = [(35, 10), (30, 40), (60, 30), (50, 50), (40, 35), (10, 40)]
# 种群大小
pop_size = 50
# 迭代次数
max_iter = 100
# 交叉概率
crossover_prob = 0.8
# 变异概率
mutation_prob = 0.1
# 初始化种群
def init_population():
population = []
for i in range(pop_size):
chromosome = []
for j in range(len(items)):
chromosome.append(random.randint(0, 1))
population.append(chromosome)
return population
# 计算适应度函数值
def fitness(chromosome):
weight = 0
value = 0
for i in range(len(chromosome)):
if chromosome[i] == 1:
weight += items[i][0]
value += items[i][1]
if weight > capacity:
value = 0
return value
# 选择操作
def selection(population):
fitness_values = [fitness(chromosome) for chromosome in population]
total_fitness = sum(fitness_values)
probabilities = [fitness_value / total_fitness for fitness_value in fitness_values]
selected_population = []
for i in range(pop_size):
selected_chromosome = random.choices(population, probabilities)[0]
selected_population.append(selected_chromosome)
return selected_population
# 交叉操作
def crossover(population):
new_population = []
for i in range(pop_size):
parent1 = population[i]
if random.random() < crossover_prob:
parent2 = random.choice(population)
crossover_point = random.randint(1, len(items) - 1)
child1 = parent1[:crossover_point] + parent2[crossover_point:]
child2 = parent2[:crossover_point] + parent1[crossover_point:]
new_population.append(child1)
new_population.append(child2)
else:
new_population.append(parent1)
return new_population
# 变异操作
def mutation(population):
for i in range(pop_size):
chromosome = population[i]
for j in range(len(items)):
if random.random() < mutation_prob:
chromosome[j] = 1 - chromosome[j]
return population
# 遗传算法求解背包问题
def genetic_algorithm():
population = init_population()
for i in range(max_iter):
population = selection(population)
population = crossover(population)
population = mutation(population)
best_chromosome = max(population, key=fitness)
best_fitness = fitness(best_chromosome)
return best_chromosome, best_fitness
# 输出最优解
best_chromosome, best_fitness = genetic_algorithm()
print("最优解:", best_chromosome)
print("最优解对应的价值:", best_fitness)
```
阅读全文
相关推荐
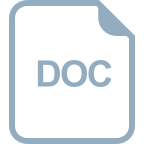
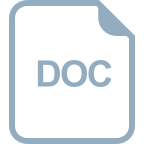
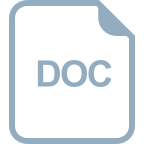
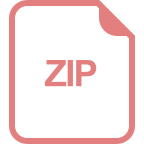

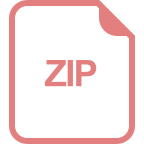
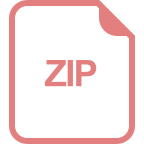
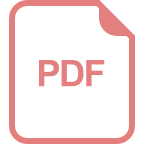
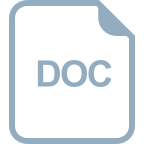
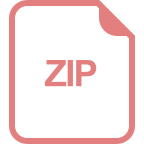
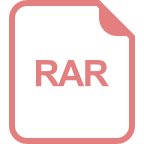
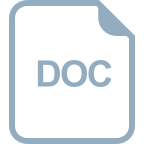
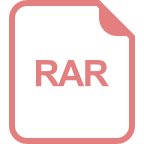
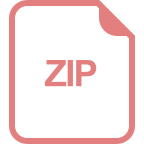
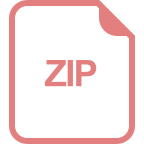

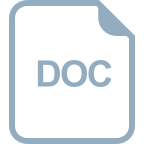