python设计计算器多功能
时间: 2024-10-18 21:05:13 浏览: 16
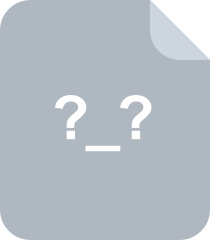
python 实现多功能科学计算器
在Python中设计一个多功能计算器,你可以创建一个类来封装加、减、乘、除等基本数学运算,并可能添加一些额外的功能如取模、幂运算以及处理用户输入错误。下面是一个简单的示例:
```python
class Calculator:
def add(self, num1, num2):
return num1 + num2
def subtract(self, num1, num2):
return num1 - num2
def multiply(self, num1, num2):
return num1 * num2
def divide(self, num1, num2):
if num2 != 0:
return num1 / num2
else:
return "Error! Division by zero is not allowed."
def modulus(self, num1, num2):
return num1 % num2
def power(self, base, exponent):
return base ** exponent
def input_and_calculate(self, operation, *args):
if operation in ['+', '-', '*', '/', '%', '^']:
try:
result = getattr(self, operation)(*args)
return result
except Exception as e:
return str(e)
else:
return "Invalid operation. Please use one of the following: +, -, *, /, %, ^"
# 使用示例
calc = Calculator()
print(calc.input_and_calculate('+', 10, 5)) # 输出:15
print(calc.input_and_calculate('/', 10, 0)) # 输出:"Error! Division by zero is not allowed."
```
在这个例子中,`Calculator`类包含了各种基本操作方法,用户可以通过传递相应的操作符和数字调用这些方法。`input_and_calculate`函数用于接收用户的输入并调用适当的方法。
阅读全文
相关推荐
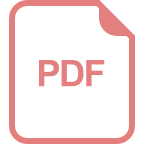
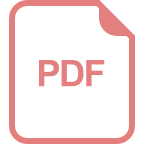
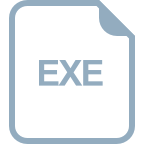
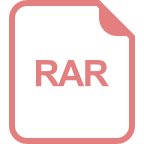
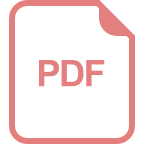
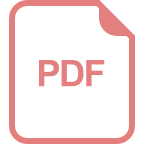
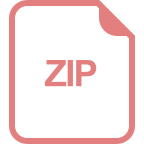
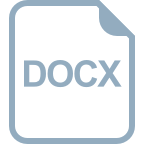
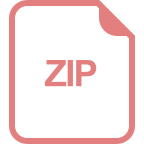
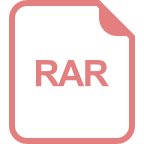
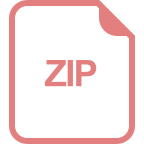
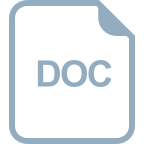
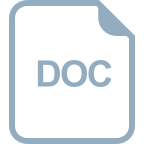
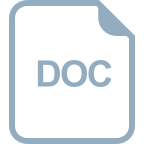



