Security框架退出登陆java代码实现
时间: 2024-10-19 14:05:58 浏览: 21
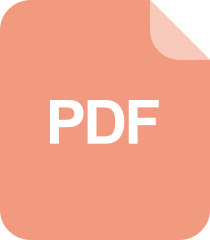
Spring security实现登陆和权限角色控制

在Java中,实现Security框架的登录注销功能通常涉及到Spring Security库的使用。以下是简单的一种实现方式:
首先,在配置文件`application.properties`或`application.yml`中,你可以设置Spring Security的相关属性,例如:
```properties
spring.security.user.name=admin
spring.security.user.password=password
```
然后,在`WebSecurityConfigurerAdapter`或`SecurityConfig`类中配置登录处理:
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private UserDetailsService userDetailsService;
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/", "/login").permitAll() // 允许访问登录页面
.anyRequest().authenticated() // 非登录请求需要认证
.and()
.formLogin()
.loginPage("/login") // 登录页面路径
.defaultSuccessUrl("/") // 登录成功后默认跳转到主页
.permitAll()
.and()
.logout() // 注销处理
.logoutSuccessUrl("/") // 注销后跳转到主页
.logoutRequestMatcher(new AntPathRequestMatcher("/logout")) // 注销链接的映射
.deleteCookies("JSESSIONID"); // 清除JSESSIONID cookie
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(userDetailsService); // 使用自定义用户服务加载用户信息
}
}
```
当用户成功登录后,可以在适当的地方调用`SecurityContextHolder.getContext().getAuthentication().getName()`获取当前登录用户的用户名,进行后续的操作。如果需要退出登录,则可以调用:
```java
// 可以在控制器或者拦截器等地方
@Autowired
private AuthenticationManager authenticationManager;
public String logout() {
try {
Authentication authentication = SecurityContextHolder.getContext().getAuthentication();
if (authentication != null) {
authentication.logout();
}
return "redirect:/login"; // 返回到登录页
} catch (Exception e) {
logger.error("Logout failed", e);
return "error"; // 返回错误页面
}
}
```
阅读全文
相关推荐
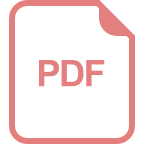
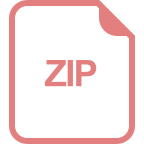
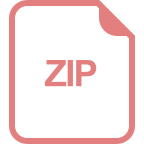
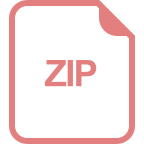
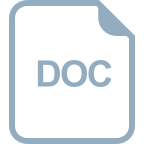
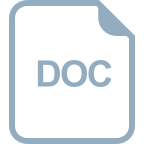
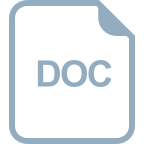
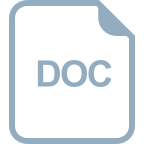
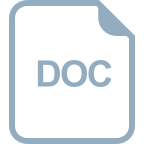
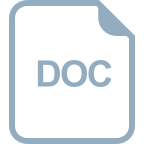
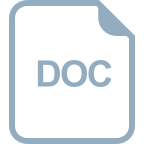
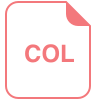





