在C#中,如何设计一个类来表示几何图形(包括矩形、圆形和三角形),并为每个图形提供两个构造方法,分别用于设置必要的属性(例如矩形的长和宽、圆的半径或三角形的边长等)。然后,请指导如何编写计算并分别输出这些图形周长和面积的方法?
时间: 2024-12-19 14:17:18 浏览: 9
在C#中,我们可以创建一个名为`Shape`的基类,作为几何形状的通用模板,并为其提供一些基本的方法,如计算周长和面积。接着,我们可以为每个具体的形状(矩形、圆形和三角形)创建派生类,实现各自的构造方法和特有的属性。
首先,我们定义基础`Shape`类:
```csharp
public abstract class Shape
{
public abstract double CalculatePerimeter();
public abstract double CalculateArea();
protected Shape(double... attributes) // 具体形状的属性将在此处设定
{
// 初始化属性
foreach (var attribute in attributes)
this.SetAttribute(attribute);
}
protected virtual void SetAttribute(double value)
{
throw new NotImplementedException("Please implement for specific shapes.");
}
}
```
接下来,为每个形状创建派生类:
1. `Rectangle` 类:
```csharp
public class Rectangle : Shape
{
private double width;
private double height;
public Rectangle(double width, double height)
: base(width, height)
{
this.width = width;
this.height = height;
}
protected override void SetAttribute(double value)
{
if (value == width || value == height)
return; // 如果是已知属性,则不做处理
throw new ArgumentException($"Invalid attribute value for rectangle: {value}");
}
public override double CalculatePerimeter()
{
return 2 * (width + height);
}
public override double CalculateArea()
{
return width * height;
}
}
```
2. `Circle` 类:
```csharp
public class Circle : Shape
{
private double radius;
public Circle(double radius)
: base(radius)
{
this.radius = radius;
}
protected override void SetAttribute(double value)
{
if (value == radius)
return; // 如果是已知属性,则不做处理
throw new ArgumentException($"Invalid attribute value for circle: {value}");
}
public override double CalculatePerimeter()
{
return 2 * Math.PI * radius;
}
public override double CalculateArea()
{
return Math.PI * radius * radius;
}
}
3. `Triangle` 类(假设是等边三角形):
```csharp
public class EquilateralTriangle : Shape
{
private double sideLength;
public EquilateralTriangle(double sideLength)
: base(sideLength)
{
this.sideLength = sideLength;
}
protected override void SetAttribute(double value)
{
if (value == sideLength)
return; // 如果是已知属性,则不做处理
throw new ArgumentException($"Invalid attribute value for equilateral triangle: {value}");
}
public override double CalculatePerimeter()
{
return 3 * sideLength;
}
public override double CalculateArea()
{
return Math.Sqrt(3) / 4 * sideLength * sideLength;
}
}
```
现在你可以创建这些形状的对象并使用它们的计算方法:
```csharp
Rectangle rectangle = new Rectangle(5, 7);
Console.WriteLine($"Rectangle perimeter: {rectangle.CalculatePerimeter()}, area: {rectangle.CalculateArea()}");
Circle circle = new Circle(4);
Console.WriteLine($"Circle perimeter: {circle.CalculatePerimeter()}, area: {circle.CalculateArea()}");
EquilateralTriangle triangle = new EquilateralTriangle(6);
Console.WriteLine($"Equilateral Triangle perimeter: {triangle.CalculatePerimeter()}, area: {triangle.CalculateArea()}");
```
阅读全文
相关推荐
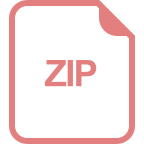
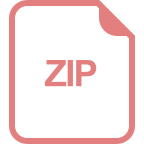
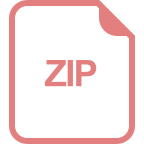
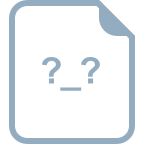
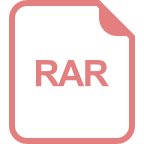
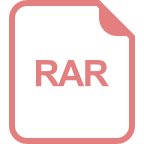
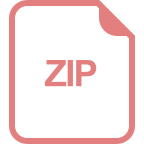
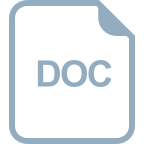

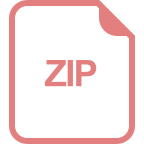
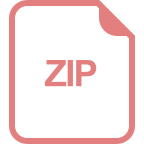