请用【java】帮我写一段快排代码,并帮我讲解下实现逻辑单价21.3元、重量12.3公斤、距离20公里
时间: 2024-11-05 13:24:17 浏览: 15
快速排序(Quick Sort)是一种常用的排序算法,其基本思想是通过一趟排序将待排序的数据分割成独立的两部分,其中一部分的所有数据都比另外一部分的所有数据都要小,然后再按此方法对这两部分数据分别进行快速排序,整个排序过程可以递归进行,以此达到整个数据变成有序序列。
以下是使用Java编写的快速排序代码:
```java
public class QuickSort {
public void quickSort(int[] arr, int low, int high) {
if (low < high) {
// 选择枢轴元素
int pivotIndex = partition(arr, low, high);
// 对枢轴左边和右边的部分进行递归排序
quickSort(arr, low, pivotIndex - 1);
quickSort(arr, pivotIndex + 1, high);
}
}
private int partition(int[] arr, int low, int high) {
int pivot = arr[high]; // 最后一个元素作为基准
int i = (low - 1); // 小于基准的元素索引
for (int j = low; j <= high - 1; j++) {
// 如果当前元素小于或等于基准
if (arr[j] <= pivot) {
i++; // 更新较小元素的索引
swap(arr, i, j);
}
}
swap(arr, i + 1, high); // 将基准移到正确的位置
return i + 1;
}
private void swap(int[] arr, int i, int j) {
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
public static void main(String[] args) {
int[] arr = {4, 2, 9, 6, 5, 1};
int n = arr.length;
QuickSort quickSortInstance = new QuickSort();
quickSortInstance.quickSort(arr, 0, n - 1);
System.out.println("Sorted array: ");
for (int i : arr) {
System.out.print(i + " ");
}
}
}
```
这个程序首先选择数组最后一个元素作为基准,然后从第一个元素开始遍历数组,如果遇到比基准小的元素就将其与i位置的元素交换,同时更新i的值。遍历完成后,基准会位于最终正确的位置,然后递归地对左右两个子数组进行同样的操作。
关于您提到的价格、重量和距离信息,这似乎与快速排序算法本身无关。如果您想实现类似功能的排序,比如基于价格的升序排列,您可以稍微修改上面的代码,将商品对象存储在一个列表里,包含价格属性,然后按照价格进行排序。对于不同类型的数值,您还可以选择其他排序算法,如基数排序等。
阅读全文
相关推荐
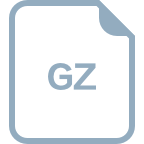
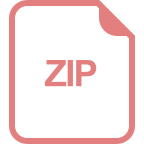
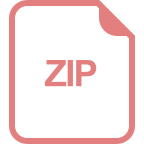
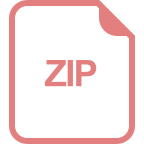
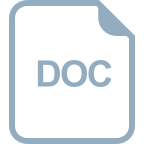
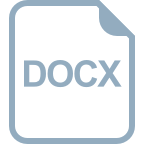
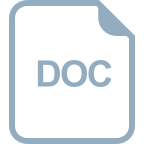
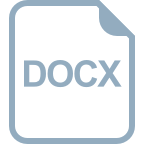
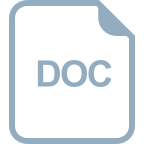
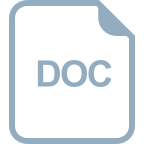
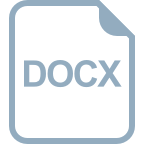
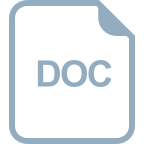
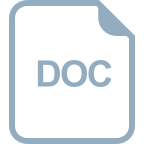