如何对接 API GET接口 写一个实例
时间: 2024-10-03 10:04:23 浏览: 33
在编程中,特别是使用像Python、JavaScript或Java这样的语言时,API(Application Programming Interface)GET接口通常用于从远程服务器获取数据而不需要发送任何数据。下面是一个简单的例子,我会展示如何使用JavaScript(Node.js环境)通过`axios`库来调用一个GET接口:
```javascript
// 引入axios库用于HTTP请求
const axios = require('axios');
// 定义需要访问的API URL
const apiUrl = 'https://api.example.com/data?key=your_api_key';
// 使用axios.get()方法发起GET请求
axios.get(apiUrl)
.then(response => {
// 请求成功,处理返回的数据
console.log('Response:', response.data);
})
.catch(error => {
// 请求失败,处理错误
console.error('Error:', error.message);
});
```
在这个例子中,你需要将`https://api.example.com/data`替换为你实际要调用的API地址,并在URL中提供必要的查询参数或认证信息(如`key`)。如果请求成功,`response.data`会包含接收到的数据;如果请求出错,会在控制台打印错误信息。
相关问题
C# 对接https://open-api.hikiot.com海康互联接口
在C#中对接海康互联(HiKiOT)的API,通常你需要遵循以下几个步骤:
1. **添加依赖**:
- 首先,确保你的项目已经安装了用于处理HTTP请求的库,如HttpClient、RestSharp或者使用NuGet包如`Microsoft.HttpClient`或第三方库如`Octokit`(如果你需要处理JSON数据)。
2. **获取API密钥**:
- 登录到海康互联开发者平台,注册并创建应用以获取API Key和Secret,这将用于身份验证。
3. **编写代码**:
```csharp
using System;
using RestSharp;
// 或者其他HTTP客户端库
public class HikiotApiClient
{
private readonly string _baseUrl = "https://open-api.hikiot.com";
private readonly string _apiKey;
private readonly string _apiSecret;
public HikiotApiClient(string apiKey, string apiSecret)
{
_apiKey = apiKey;
_apiSecret = apiSecret;
}
public async Task<string> GetDeviceData(string deviceId)
{
var client = new RestClient(_baseUrl);
var request = new RestRequest(Method.GET);
request.AddParameter("device_id", deviceId);
// 添加API认证信息
var auth = new HttpBasicAuth(_apiKey, _apiSecret);
request.Authenticator = auth;
request.Resource = "/v1/devices/{deviceId}/data"; // 替换{deviceId}为你设备的实际ID
var response = await client.ExecuteAsync(request);
return await response.Content.ReadAsStringAsync();
}
}
```
4. **发起请求**:
- 创建`HikiotApiClient`实例,并通过设备ID调用`GetDeviceData`方法来获取数据。
5. **处理错误和异常**:
- 确保检查`ExecuteAsync`返回的`IRestResponse`的状态码,处理可能出现的网络问题、授权失败等。
c# 对接webservice接口开发
在C#中,对接Web服务接口通常涉及到SOAP(Simple Object Access Protocol)或RESTful API的开发。以下是步骤和一些关键概念:
1. **添加命名空间**:首先,在C#项目中添加对System.Web.Services或System.Net.Http命名空间的引用,这取决于你要使用的协议(SOAP还是REST)。
2. **创建Service Reference**:
- 对于SOAP Web Service:右键点击解决方案 -> 添加 -> 新项 -> WCF服务参考 -> 选择wsdl文件或URL,生成代理类。
- 对于RESTful API:可以使用HttpClient或第三方库如RestSharp直接发送HTTP请求,不需要创建服务引用。
3. **编写客户端代码**:
- SOAP Web Service:使用生成的`Service1.cs`等类,实例化服务并调用其方法,传递参数。
```csharp
Service1 service = new Service1();
string response = service.ServiceMethod(parameters);
```
- RESTful API:使用HttpClient发送POST、GET等请求,并处理响应。
```csharp
HttpClient client = new HttpClient();
HttpResponseMessage httpResponse = await client.GetAsync("api/resource");
string responseBody = await httpResponse.Content.ReadAsStringAsync();
```
4. **错误处理**:别忘了处理可能出现的异常,包括网络连接失败、API返回的状态码不是200等。
5. **调试和测试**:使用工具如Visual Studio的自带工具,或者Postman等API测试工具检查请求和响应是否正确。
阅读全文
相关推荐




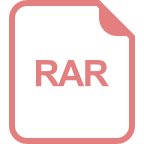











