C语言输入年份显示当年日历4行
时间: 2024-12-28 07:40:21 浏览: 13
在C语言中,要实现在用户输入年份后显示该年的日历,你可以使用标准库函数`cal()`。这个函数可以获取指定年份的月份的日历,并打印出来。以下是简单的步骤:
```c
#include <stdio.h>
#include <time.h>
int main() {
int year;
// 获取用户输入的年份
printf("请输入年份: ");
scanf("%d", &year);
// 检查是否为闰年
int is_leap = (year % 4 == 0 && (year % 100 != 0 || year % 400 == 0));
// 创建结构体tm并设置年、月
struct tm date = {0};
date.tm_year = year - 1900; // 因为ctime函数偏移了1900年
date.tm_mon = 0; // 从一月开始
// 循环遍历一年中的所有月份
for(int i = 0; i <= 11; ++i) {
// 设置月份
date.tm_mon = i;
// 转换为时间结构并输出日历
time_t t = mktime(&date);
char calendar[80];
strftime(calendar, sizeof(calendar), "%a %b %e %Y", &date); // 星期几 + 月份 + 日 + 年份
if(is_leap && i == 2 && date.tm_mday > 29) continue; // 如果是闰年的二月,跳过非法日期
printf("%s\n", calendar); // 打印日历行
}
return 0;
}
```
当你运行这个程序并输入年份后,它会打印出对应年份每个月的日历。注意,`strftime()` 函数用于格式化时间,而 `mktime()` 函数则将 `struct tm` 转换为时间戳。
阅读全文
相关推荐


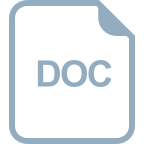

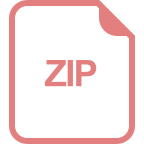




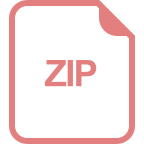








