html 可移动悬浮按钮
时间: 2024-10-22 14:24:18 浏览: 46
HTML 中的可移动悬浮按钮通常通过 CSS 和 JavaScript 实现。你可以创建一个基本的 HTML 按钮元素,并添加样式使其浮动。CSS 的 `position` 属性可以设置为 `fixed` 来使元素相对于浏览器窗口固定定位,而 `top`, `right`, `bottom`, 或 `left` 属性控制其在屏幕上的位置。例如:
```html
<!DOCTYPE html>
<html lang="zh">
<head>
<style>
.float-btn {
position: fixed;
top: 20px; /* 鼠标距离顶部的距离 */
right: 20px; /* 鼠标距离右侧的距离 */
background-color: #337ab7;
color: white;
padding: 10px 20px;
border: none;
cursor: pointer;
}
</style>
</head>
<body>
<button class="float-btn">点击我</button>
</body>
</html>
```
JavaScript 可以用于响应用户的交互,比如点击事件,或者动态调整按钮的位置。然而,在现代前端开发中,更推荐使用响应式设计框架如 Bootstrap,它内置了易于使用的浮动导航组件。
相关问题
html可移动的悬浮按钮,js仿苹果悬浮可拖拽按钮,并且点击展开效果
要实现一个可移动的悬浮按钮,并且点击后有展开效果,可以使用 HTML、CSS 和 JavaScript。下面是一个简单的示例代码:
HTML 代码:
```
<div id="floatBtn">
<div class="btn-icon">按钮</div>
<div class="btn-panel">
<ul>
<li>选项一</li>
<li>选项二</li>
<li>选项三</li>
</ul>
</div>
</div>
```
CSS 代码:
```
#floatBtn {
position: fixed;
bottom: 20px;
right: 20px;
z-index: 9999;
}
.btn-icon {
background-color: #4CAF50;
color: white;
border: none;
border-radius: 50%;
padding: 15px;
font-size: 24px;
cursor: pointer;
}
.btn-panel {
display: none;
position: absolute;
bottom: 70px;
right: 0;
background-color: white;
border: 1px solid #ccc;
padding: 10px;
}
.btn-panel ul {
list-style: none;
margin: 0;
padding: 0;
}
.btn-panel ul li {
padding: 5px;
cursor: pointer;
}
.btn-panel ul li:hover {
background-color: #f5f5f5;
}
```
JavaScript 代码:
```
var floatBtn = document.getElementById("floatBtn");
var btnIcon = floatBtn.querySelector(".btn-icon");
var btnPanel = floatBtn.querySelector(".btn-panel");
// 点击按钮展开/收起面板
btnIcon.addEventListener("click", function() {
if (btnPanel.style.display === "none") {
btnPanel.style.display = "block";
} else {
btnPanel.style.display = "none";
}
});
// 拖拽按钮
var isDragging = false;
var offsetX = 0;
var offsetY = 0;
btnIcon.addEventListener("mousedown", function(e) {
isDragging = true;
offsetX = e.clientX - floatBtn.offsetLeft;
offsetY = e.clientY - floatBtn.offsetTop;
});
document.addEventListener("mousemove", function(e) {
if (isDragging) {
var x = e.clientX - offsetX;
var y = e.clientY - offsetY;
floatBtn.style.left = x + "px";
floatBtn.style.top = y + "px";
}
});
document.addEventListener("mouseup", function() {
isDragging = false;
});
```
这段代码实现了一个在页面右下角的悬浮按钮,点击按钮可以展开一个带有选项的面板,同时也实现了可拖拽的效果。
h5可拖动悬浮按钮_js实现悬浮窗效果(支持拖动)
以下是一个简单的实现可拖动悬浮按钮的代码示例,使用 JavaScript 和 HTML5 的 drag-and-drop API:
HTML 代码:
```html
<div id="floating-button" draggable="true">
<span>按钮</span>
</div>
```
CSS 代码:
```css
#floating-button {
position: fixed;
bottom: 20px;
right: 20px;
width: 100px;
height: 50px;
background-color: #4CAF50;
color: white;
border-radius: 10px;
text-align: center;
line-height: 50px;
cursor: pointer;
}
```
JavaScript 代码:
```javascript
const floatingButton = document.getElementById('floating-button');
// 当用户开始拖动悬浮按钮时
floatingButton.addEventListener('dragstart', function (event) {
// 记录起始坐标
event.dataTransfer.setData('startX', event.clientX);
event.dataTransfer.setData('startY', event.clientY);
});
// 当用户正在拖动悬浮按钮时
floatingButton.addEventListener('drag', function (event) {
// 计算偏移量
const startX = event.dataTransfer.getData('startX');
const startY = event.dataTransfer.getData('startY');
const offsetX = event.clientX - startX;
const offsetY = event.clientY - startY;
// 移动悬浮按钮
const initialX = parseInt(window.getComputedStyle(floatingButton).getPropertyValue('right'));
const initialY = parseInt(window.getComputedStyle(floatingButton).getPropertyValue('bottom'));
floatingButton.style.right = `${initialX - offsetX}px`;
floatingButton.style.bottom = `${initialY - offsetY}px`;
});
```
这段代码创建了一个可拖动的悬浮按钮,并且允许用户通过鼠标拖动按钮移动它。当用户开始拖动按钮时,我们记录起始坐标,然后当用户正在拖动按钮时,我们计算当前位置和起始位置之间的偏移量,并将悬浮按钮相应地移动。
阅读全文
相关推荐
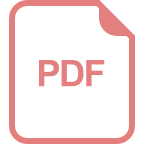
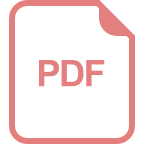
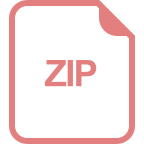
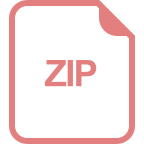
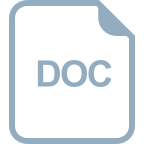
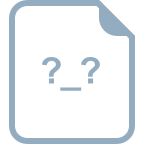
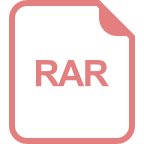
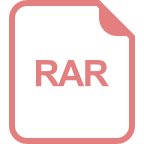
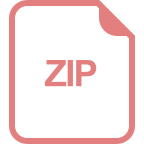
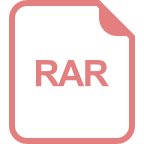
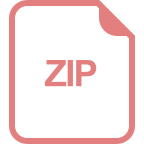



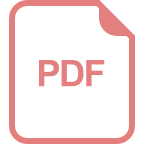
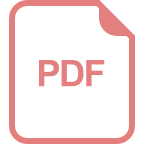