vue实现文件上传功能加上springboot
时间: 2024-10-26 18:17:41 浏览: 33
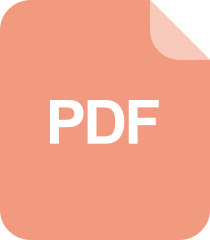
springboot+vue实现文件上传下载
Vue.js 实现文件上传功能结合 Spring Boot 主要有以下几个步骤:
1. **前端 Vue 部分**:
- 使用 `vue-file-input` 或 `vue-quill-image-uploader` 这样的第三方库来添加文件输入组件,如 `<el-upload>` 或者 `<vue-file-preview>`.
- 设置事件处理器,当用户选择文件后,发送请求到服务器。可以利用 Vue 的 `axios` 或者官方推荐的 `@vue/cli-plugin-apollo` (如果使用 GraphQL) 发送 POST 请求。
```html
<template>
<div>
<el-upload :action="uploadUrl" :on-success="handleSuccess" :file-list="files">
<i class="el-icon-plus"></i>
<span slot="tip">点击上传</span>
</el-upload>
</div>
</template>
<script>
import axios from 'axios';
export default {
data() {
return {
files: [],
uploadUrl: '/api/upload', // Spring Boot REST API 接口地址
};
},
methods: {
handleSuccess(response) {
console.log('文件上传成功', response);
this.files.push(response.data); // 将服务器返回的新文件信息添加到列表
}
}
};
</script>
```
2. **后端 Spring Boot 部分**:
- 创建一个 Spring 控制器,处理文件上传请求。Spring 提供了 `MultipartFile` 对象用于处理文件,你可以将其保存到本地存储、数据库或者上传到云服务。
- 可能需要设置 multipart/form-data 格式作为请求内容类型,并配置文件大小限制等参数。
```java
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.multipart.MultipartFile;
import java.io.File;
import java.io.IOException;
@RestController
public class FileUploadController {
@PostMapping("/api/upload")
public ResponseEntity<String> handleFileUpload(@RequestParam("file") MultipartFile file) throws IOException {
if (!file.isEmpty()) {
String filename = file.getOriginalFilename();
byte[] bytes = file.getBytes();
// 拷贝文件到服务器目录
File targetFile = new File("uploads/" + filename);
Files.copy(file.getInputStream(), targetFile.toPath());
return ResponseEntity.ok("文件 " + filename + " 已上传");
} else {
return ResponseEntity.badRequest().body("文件为空");
}
}
}
```
阅读全文
相关推荐
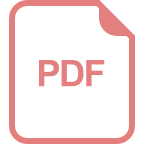
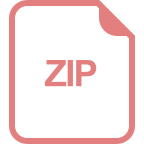
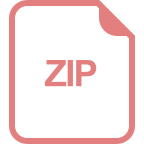
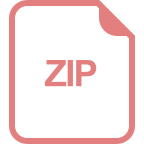
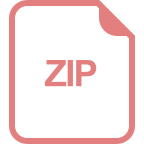
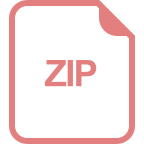
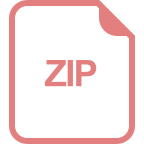
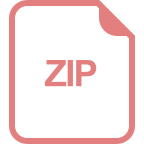
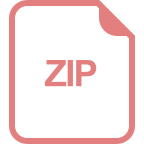
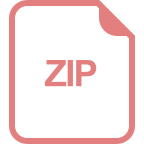
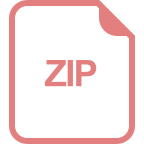
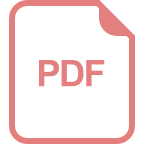
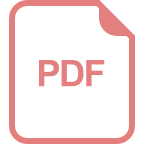
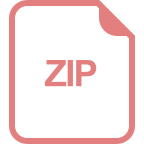
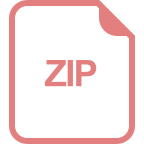
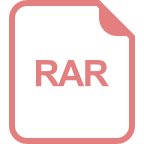