.NET读写config配置文件
时间: 2025-01-08 14:42:42 浏览: 1
### .NET 中读写 Config 文件的方法
在.NET框架中,`app.config` 或 `web.config` 是特殊的配置文件,无法像一般 XML 文件那样简单地通过序列化方式处理。对于这些特定类型的配置文件,有多种方法可以实现其读取和修改操作。
#### 使用 ConfigurationManager 类读取配置项
为了访问应用程序中的设置部分,可利用 `System.Configuration` 命名空间下的 `ConfigurationManager` 类来获取应用级别的配置数据[^1]:
```csharp
string appSettingValue = ConfigurationManager.AppSettings["MyAppSettingKey"];
```
此代码片段展示了如何从 `<appSettings>` 节点检索键值对形式的应用程序设定。
#### 修改现有配置文件并保存更改
当需要更新现有的配置条目时,则可以通过打开当前执行文件对应的配置实例来进行编辑,并最终调用 Save 方法持久化变更[^2]:
```csharp
using System;
using System.Configuration;
class Program {
static void Main(string[] args){
Configuration config = ConfigurationManager.OpenExeConfiguration(ConfigurationUserLevel.None);
// 更新 AppSettings 的某个 key-value 对
string myKeyValue = "New Value";
config.AppSettings.Settings["SomeKey"].Value = myKeyValue;
// 将更改后的配置保存回磁盘上的配置文件
config.Save();
Console.WriteLine($"Updated value of SomeKey to '{myKeyValue}'");
}
}
```
上述例子说明了怎样定位到指定的配置节(这里是 `appSettings`),并对其中的内容作出调整;之后再把新的状态存入原始位置。
#### 创建自定义配置节点及其管理
如果项目需求涉及到更复杂的结构化信息存储于配置文件内,那么应该考虑设计自己的配置元素、集合以及相应的处理器类。这通常涉及继承某些基类如 `ConfigurationElement`, `ConfigurationSectionGroup`, 和/或 `ConfigurationSection` 来构建层次化的配置模型[^3].
下面给出了一段创建自定义配置区段的例子:
```xml
<configuration>
<configSections>
<!-- 定义一个新的 section -->
<section name="customConfig" type="CustomConfigSection, MyAssembly"/>
</configSections>
<!-- 实际使用的 customConfig section -->
<customConfig settingName="value">
...
</customConfig>
</configuration>
```
配合 C# 端代码如下所示:
```csharp
public class CustomConfigSection : ConfigurationSection{
[ConfigurationProperty("settingName", IsRequired=true)]
public string SettingName => (string)this["settingName"];
// 可继续添加更多属性...
}
// 访问该自定义配置区段
var customConfig = (CustomConfigSection)ConfigurationManager.GetSection("customConfig");
Console.WriteLine(customConfig.SettingName);
```
这段示范解释了如何注册新类型的配置区块至全局配置表单之中,并且提供了相应 API 接口供后续编程逻辑调用。
阅读全文
相关推荐



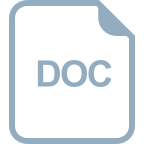



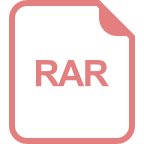








