如何在Python程序中将图片文件添加到项目根目录下?
时间: 2024-10-23 10:19:58 浏览: 16
在Python中,你可以使用内置的`os`模块来操作文件系统,包括将图片文件添加到项目的根目录下。以下是简单的步骤:
1. 首先,你需要确认图片文件已经存在。如果你有一个本地文件路径,可以先检查它是否存在。
```python
import os
image_path = "path_to_your_image.jpg" # 替换为你图片的实际路径
if not os.path.isfile(image_path):
print(f"图片文件 {image_path} 不存在.")
else:
```
2. 使用`os.makedirs`函数创建项目的根目录,如果它还没有存在。这一步不是必需的,但如果项目根目录不存在,会自动创建。
```python
root_dir = "./" # 项目根目录,假设在当前工作目录下
if not os.path.exists(root_dir):
os.makedirs(root_dir)
3. 确定目录已创建后,你可以使用`shutil.copyfile`或`shutil.move`方法将图片复制到根目录中。这里我们使用`copyfile`示例:
```python
destination_path = os.path.join(root_dir, "image.jpg") # 新的目标路径,包含文件名
try:
shutil.copyfile(image_path, destination_path)
except Exception as e:
print(f"复制图片时出错: {e}")
4. 最后,你可以检查图片是否成功移动到新位置:
```python
print(f"图片 '{image_path}' 已经成功添加到项目根目录: {destination_path}")
```
记得替换`image_path`为你的实际图片路径。完成上述步骤后,图片就添加到项目根目录了。
阅读全文
相关推荐
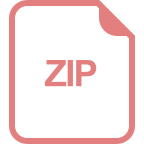
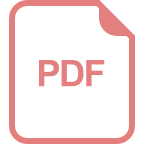
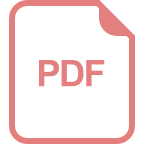
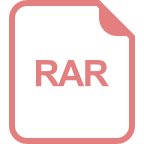
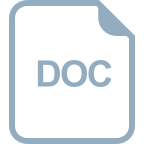
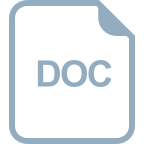
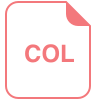
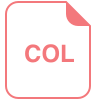
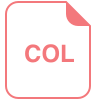
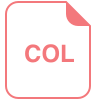
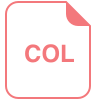
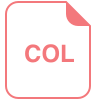
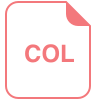
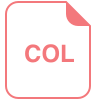
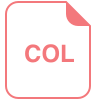

