如何用c语言实现一个顺序线性表?
时间: 2024-09-19 16:12:39 浏览: 21
在C语言中,可以使用数组或链表数据结构来实现一个简单的顺序线性表。这里我们以单向链表为例,因为链表可以动态地添加或删除元素,更适应动态大小的需求。
首先,我们需要定义一个节点结构体,包含一个数据域和指向下一个节点的指针:
```c
typedef struct Node {
int data; // 数据域,存储元素值
struct Node* next; // 指针,指向下一个节点
} ListNode;
```
接着,创建一个函数用于创建链表的头部节点:
```c
ListNode* createList(int value) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
if (!newNode) {
printf("Memory allocation failed.\n");
return NULL;
}
newNode->data = value;
newNode->next = NULL;
return newNode;
}
```
然后,我们可以定义插入元素到链表中的函数:
```c
void insertNode(ListNode** head, int value) {
ListNode* newNode = createList(value);
if (*head == NULL) {
*head = newNode;
} else {
ListNode* current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
}
```
最后,如果需要遍历链表,可以用递归或迭代的方式实现:
```c
void traverseList(ListNode* head) {
if (head == NULL) {
printf("List is empty.\n");
return;
}
printf("%d ", head->data); // 打印节点值
traverseList(head->next); // 递归访问下一个节点
}
```
使用示例:
```c
int main() {
ListNode* head = NULL;
insertNode(&head, 10);
insertNode(&head, 20);
insertNode(&head, 30);
printf("Linear list: ");
traverseList(head);
return 0;
}
```
相关推荐
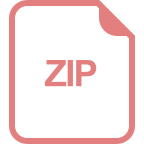
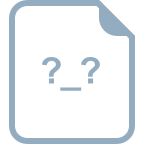
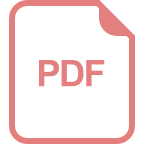














