C#实现异地文件复制
时间: 2024-09-11 21:01:31 浏览: 42
在C#中实现异地文件复制通常涉及到I/O操作和网络通信。你可以使用`System.IO.File.Copy`方法结合`System.Net.Http`或者`System.Net.WebClient`来跨域复制文件。以下是基本的步骤:
1. **使用`File.Copy`和`WebClient`**:
```csharp
using System;
using System.IO;
using System.Net;
public static void CopyRemoteFile(string sourceUrl, string destinationPath)
{
try
{
// 创建WebClient实例
WebClient client = new WebClient();
// 下载文件到内存流
byte[] bytes = client.DownloadData(sourceUrl);
// 将数据写入本地文件
using (FileStream fs = File.Create(destinationPath))
{
fs.Write(bytes, 0, bytes.Length);
}
Console.WriteLine($"文件已从{sourceUrl}复制到{destinationPath}");
}
catch (Exception ex)
{
Console.WriteLine($"文件复制失败: {ex.Message}");
}
}
```
2. **使用`HttpClient`**:
```csharp
using System;
using System.Net.Http;
using System.Threading.Tasks;
public async static Task CopyRemoteFileAsync(string sourceUrl, string destinationPath)
{
using HttpClient httpClient = new HttpClient();
try
{
HttpResponseMessage response = await httpClient.GetAsync(sourceUrl);
response.EnsureSuccessStatusCode();
byte[] bytes = await response.Content.ReadAsByteArrayAsync();
await File.WriteAllBytesAsync(destinationPath, bytes);
Console.WriteLine($"文件已从{sourceUrl}复制到{destinationPath}");
}
catch (Exception ex)
{
Console.WriteLine($"文件复制失败: {ex.Message}");
}
}
```
记得处理可能出现的异常,并确保源地址可以正常访问。
阅读全文
相关推荐
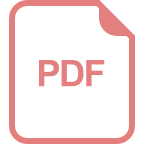
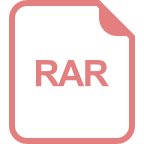
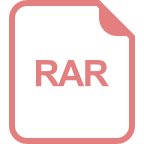
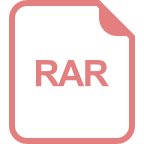
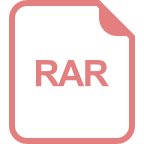
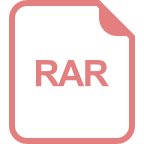
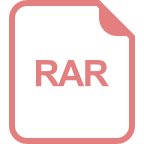
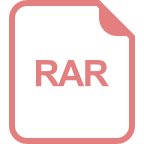
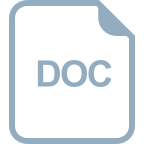
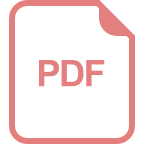
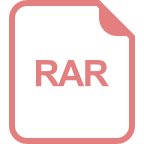
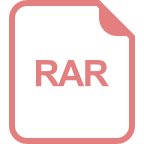
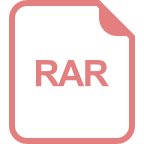
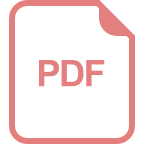
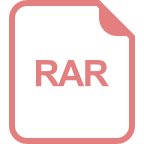
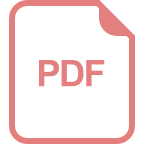
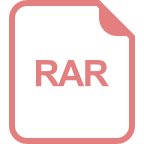