qt中,每连接一次tcp就往文件中写一次时间的代码
时间: 2024-12-25 17:34:54 浏览: 0
在Qt中,如果你想要每次成功连接TCP后都向文件中写入当前的时间,你可以使用QIODevice、QTcpSocket以及QDateTime等类来实现。这里是一个简单的示例代码片段:
```cpp
#include <QObject>
#include <QTcpSocket>
#include <QFile>
#include <QDateTime>
class TcpConnectionHandler : public QObject
{
Q_OBJECT
public:
explicit TcpConnectionHandler(QObject *parent = nullptr)
: QObject(parent)
{
connect(&tcpSocket, &QTcpSocket::connected, this, &TcpConnectionHandler::onConnected);
connect(&tcpSocket, &QTcpSocket::disconnected, this, &TcpConnectionHandler::onDisconnected);
}
private slots:
void onConnected()
{
QDateTime currentTime = QDateTime::currentDateTime();
QString dateTimeStr = currentTime.toString("yyyy-MM-dd HH:mm:ss");
QFile file("time_log.txt");
if (file.open(QIODevice::Append | QIODevice::Text)) {
QTextStream out(&file);
out << "Connected at: " << dateTimeStr << "\n";
file.close();
qDebug() << "Time logged: " << dateTimeStr;
} else {
qWarning() << "Failed to open file for writing.";
}
}
void onDisconnected()
{
// Write disconnection time here or handle accordingly
}
signals:
// If needed, you can emit a signal when connected or disconnected
};
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
TcpConnectionHandler handler;
QTcpServer server(&handler);
server.listen(QHostAddress::Any, 12345);
return app.exec();
}
```
在这个例子中,我们创建了一个`TcpConnectionHandler`类,当TCP连接建立时,会记录当前时间并追加到`time_log.txt`文件中。如果文件打开失败,会在控制台输出警告信息。
阅读全文
相关推荐
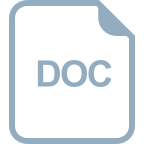
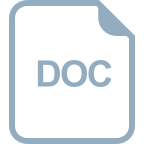
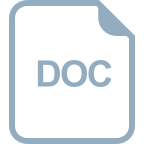
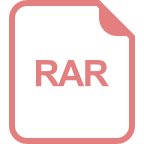
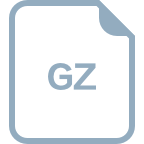
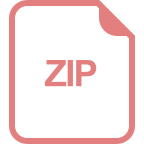
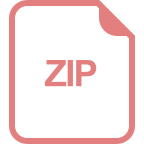
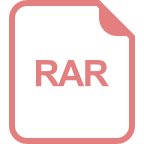
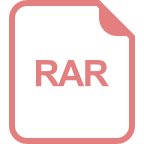









