os.makedirs(desktop)
时间: 2023-10-12 07:55:24 浏览: 149
`os.makedirs(desktop)` 是一个Python中的函数调用,用于创建一个目录。
在这个特定的调用中,`desktop` 是一个参数,表示要创建的目录的路径。然而,这个代码是不正确的,因为 `desktop` 并没有被正确地引用。
正确的用法是使用引号将路径字符串包围起来,如下所示:
```python
import os
desktop = "path/to/desktop" # 请将"path/to/desktop"替换为你想要创建目录的路径
os.makedirs(desktop)
```
通过这个代码,你可以在指定的路径上创建一个名为 `desktop` 的目录。
请确保提供正确的路径,并在运行代码之前导入 `os` 模块。
如果还有其他问题,请随时提问。
相关问题
这段代码在运行时import SimpleITK as sitkimport numpy as npimport os# 设置文件路径data_path = 'C:/Users/Administrator/Desktop/LiTS2017/'save_path = 'C:/Users/Administrator/Desktop/2D-LiTS2017/'if not os.path.exists(save_path): os.makedirs(save_path)# 定义函数将3D图像保存为2D的.png格式def save_image_as_png(image, save_folder, name_prefix): for i in range(image.shape[2]): slice = np.squeeze(image[:, :, i]) slice = slice.astype(np.float32) slice *= 255.0/slice.max() slice = slice.astype(np.uint8) save_name = os.path.join(save_folder, name_prefix + '_' + str(i) + '.png') sitk.WriteImage(sitk.GetImageFromArray(slice), save_name)# 读取Training Batch 1中的图像image_path = os.path.join(data_path, 'Training Batch 1/volume-0.nii')image = sitk.ReadImage(image_path)image_array = sitk.GetArrayFromImage(image)save_folder = os.path.join(save_path, 'image')if not os.path.exists(save_folder): os.makedirs(save_folder)save_image_as_png(image_array, save_folder, 'img')# 读取Training Batch 2中的标签label_path = os.path.join(data_path, 'Training Batch 2/segmentation-0.nii')label = sitk.ReadImage(label_path)label_array = sitk.GetArrayFromImage(label)# 将标签转换为灰度图并保存label_array[label_array == 1] = 128label_array[label_array == 2] = 255save_folder = os.path.join(save_path, 'mask')if not os.path.exists(save_folder): os.makedirs(save_folder)save_image_as_png(label_array, save_folder, 'mask')会出现RuntimeWarning: divide by zero encountered in true_divide slice *= 255.0/slice.max()这种情况,修复它
这个 RuntimeWarning 出现的原因是可能存在某些切片上所有像素都是 0,导致在计算 `slice.max()` 时出现了除以 0 的情况。解决这个问题的方法是在计算 `slice.max()` 之前,先判断 `slice` 是否全为 0,如果是,则将 `slice.max()` 设为 1。修改代码如下:
```python
def save_image_as_png(image, save_folder, name_prefix):
for i in range(image.shape[2]):
slice = np.squeeze(image[:, :, i])
slice = slice.astype(np.float32)
if slice.max() == 0:
max_val = 1
else:
max_val = slice.max()
slice *= 255.0/max_val
slice = slice.astype(np.uint8)
save_name = os.path.join(save_folder, name_prefix + '_' + str(i) + '.png')
sitk.WriteImage(sitk.GetImageFromArray(slice), save_name)
```
这样修改后,即使出现某些切片上所有像素都是 0 的情况,也不会出现除以 0 的错误了。
代码import os import numpy as np import nibabel as nib from PIL import Image # 创建保存路径 save_path = 'C:/Users/Administrator/Desktop/2D-LiTS2017' if not os.path.exists(save_path): os.makedirs(save_path) if not os.path.exists(os.path.join(save_path, 'image')): os.makedirs(os.path.join(save_path, 'image')) if not os.path.exists(os.path.join(save_path, 'label')): os.makedirs(os.path.join(save_path, 'label')) # 加载数据集 data_path = 'D:/BaiduNetdiskDownload/LiTS2017' img_path = os.path.join(data_path, 'Training Batch 1') label_path = os.path.join(data_path, 'Training Batch 2') # 转换图像 for file in sorted(os.listdir(img_path)): if file.endswith('.nii'): img_file = os.path.join(img_path, file) img = nib.load(img_file).get_fdata() img = np.transpose(img, (2, 0, 1)) # 转换为z, x, y for i in range(img.shape[0]): img_slice = img[i, :, :] img_slice = (img_slice - np.min(img_slice)) / (np.max(img_slice) - np.min(img_slice)) * 255 # 归一化到0-255 img_slice = img_slice.astype(np.uint8) img_slice = np.stack([img_slice]*3, axis=2) # 转换为三通道图像 img_name = file[:-4] + '' + str(i).zfill(3) + '.png' img_file_save = os.path.join(save_path, 'image', img_name) Image.fromarray(img_slice).save(img_file_save) # 转换标签 for file in sorted(os.listdir(label_path)): if file.endswith('.nii'): label_file = os.path.join(label_path, file) label = nib.load(label_file).get_fdata() label = np.transpose(label, (2, 0, 1)) # 转换为z, x, y for i in range(label.shape[0]): label_slice = label[i, :, :] label_slice[label_slice == 1] = 255 # 肝脏灰度值设为255 label_slice[label_slice == 2] = 128 # 肝脏肿瘤灰度值设为128 label_slice = label_slice.astype(np.uint8) label_name = file[:-4] + '' + str(i).zfill(3) + '.png' label_file_save = os.path.join(save_path, 'label', label_name) Image.fromarray(label_slice).save(label_file_save)出现scaled = scaled.astype(np.promote_types(scaled.dtype, dtype), copy=False) MemoryError错误,怎么修改?给出完整代码
这个错误通常意味着你的计算机没有足够的内存来处理数组。可能需要减少转换图像的尺寸或者使用更高效的算法。以下是一个可能的修改建议:
```python
import os
import numpy as np
import nibabel as nib
from PIL import Image
# 创建保存路径
save_path = 'C:/Users/Administrator/Desktop/2D-LiTS2017'
if not os.path.exists(save_path):
os.makedirs(save_path)
if not os.path.exists(os.path.join(save_path, 'image')):
os.makedirs(os.path.join(save_path, 'image'))
if not os.path.exists(os.path.join(save_path, 'label')):
os.makedirs(os.path.join(save_path, 'label'))
# 加载数据集
data_path = 'D:/BaiduNetdiskDownload/LiTS2017'
img_path = os.path.join(data_path, 'Training Batch 1')
label_path = os.path.join(data_path, 'Training Batch 2')
# 转换图像
for file in sorted(os.listdir(img_path)):
if file.endswith('.nii'):
img_file = os.path.join(img_path, file)
img = nib.load(img_file).get_fdata()
img = np.transpose(img, (2, 0, 1)) # 转换为z, x, y
for i in range(img.shape[0]):
img_slice = img[i, :, :]
img_slice = (img_slice - np.min(img_slice)) / (np.max(img_slice) - np.min(img_slice)) * 255 # 归一化到0-255
img_slice = img_slice.astype(np.uint8)
img_slice = np.stack([img_slice]*3, axis=2) # 转换为三通道图像
#减少图像大小
img_slice = np.array(Image.fromarray(img_slice).resize((512, 512), resample=Image.BICUBIC))
img_name = file[:-4] + '' + str(i).zfill(3) + '.png'
img_file_save = os.path.join(save_path, 'image', img_name)
Image.fromarray(img_slice).save(img_file_save)
# 转换标签
for file in sorted(os.listdir(label_path)):
if file.endswith('.nii'):
label_file = os.path.join(label_path, file)
label = nib.load(label_file).get_fdata()
label = np.transpose(label, (2, 0, 1)) # 转换为z, x, y
for i in range(label.shape[0]):
label_slice = label[i, :, :]
label_slice[label_slice == 1] = 255 # 肝脏灰度值设为255
label_slice[label_slice == 2] = 128 # 肝脏肿瘤灰度值设为128
label_slice = label_slice.astype(np.uint8)
label_name = file[:-4] + '' + str(i).zfill(3) + '.png'
label_file_save = os.path.join(save_path, 'label', label_name)
Image.fromarray(label_slice).save(label_file_save)
```
这里我们使用了 `PIL.Image` 中的 `resize()` 函数来减少图像大小。`resample=Image.BICUBIC` 用于指定插值方法,这里使用了 Bicubic 插值。这个方法可以保留图像的细节,并且可以减少内存使用。你也可以尝试使用其他插值方法。
阅读全文
相关推荐
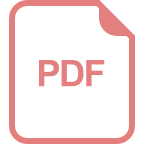
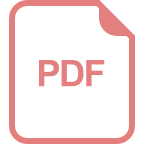
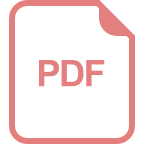










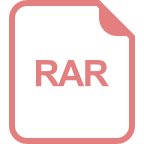
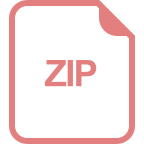
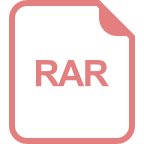
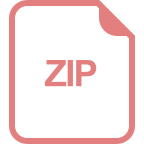