mybatis拦截器如何只拦截特定的mapper方法,被拦截的方法支持运行中修改
时间: 2024-02-06 15:02:29 浏览: 328
要实现只拦截特定的Mapper方法,并且被拦截的方法支持运行时修改,您可以使用动态代理来实现。
首先,创建一个实现了`InvocationHandler`接口的代理类,然后在该类中实现需要的拦截逻辑。
下面是一个示例代码:
```java
public class MyMapperProxy implements InvocationHandler {
private Object target;
public MyMapperProxy(Object target) {
this.target = target;
}
@Override
public Object invoke(Object proxy, Method method, Object[] args) throws Throwable {
// 获取方法所在的Mapper接口类
Class<?> mapperInterface = method.getDeclaringClass();
if (mapperInterface == YourMapper.class && method.getName().equals("yourMethod")) {
// 执行拦截逻辑
// ...
}
return method.invoke(target, args);
}
}
```
在上述示例中,通过判断`method`所在的Mapper接口类和方法名来确定是否执行拦截逻辑。您可以根据实际需求自定义拦截器的逻辑。
然后,在MyBatis的配置中使用该代理类创建Mapper的动态代理对象。
```java
@Configuration
public class MyBatisConfig {
@Autowired
private DataSource dataSource;
@Bean
public YourMapper yourMapper() {
return (YourMapper) Proxy.newProxyInstance(
YourMapper.class.getClassLoader(),
new Class[]{YourMapper.class},
new MyMapperProxy(new YourMapperImpl(dataSource)) // YourMapperImpl是实际的Mapper实现类
);
}
}
```
在上述代码中,`MyMapperProxy`作为动态代理类,将实际的Mapper实现类`YourMapperImpl`作为目标对象传入。
这样,只有特定的Mapper方法会被拦截,并且可以在运行时修改拦截逻辑。您可以根据需要在代理类的`invoke()`方法中实现相应的逻辑。注意,需要将`YourMapper`替换为您实际的Mapper接口类,并根据需要修改拦截判断条件。
阅读全文
相关推荐
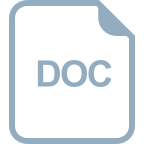
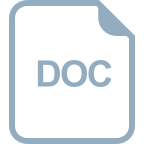
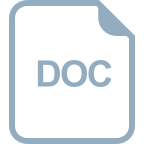





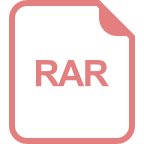
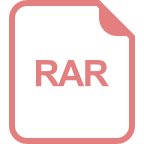
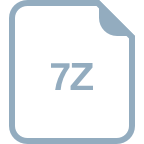
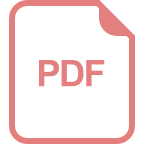
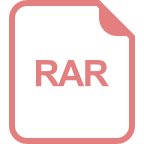
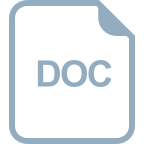




