std::unordered_map<std::string, std::shared_ptr<AbstractEdgeFindCategories>> algorithmCategories;对algorithmCategories添加元素和删除元素的函数
时间: 2024-09-15 09:04:07 浏览: 58
在C++中,`std::unordered_map` 是一种关联容器,它使用哈希表实现,提供常数时间复杂度的查找、插入和删除操作。`std::unordered_map<std::string, std::shared_ptr<AbstractEdgeFindCategories>> algorithmCategories` 定义了一个键值对映射,其中键是字符串类型,值是指向 `AbstractEdgeFindCategories` 类型对象的智能指针。
为了添加元素,你可以创建一个新的 `AbstractEdgeFindCategories` 对象,并将其包装成 `std::shared_ptr` 然后使用 `insert()` 函数:
```cpp
void addAlgorithmCategory(const std::string& categoryName, AbstractEdgeFindCategories* edgeCategory) {
std::shared_ptr<AbstractEdgeFindCategories> ptr(edgeCategory);
algorithmCategories.insert({categoryName, ptr});
}
```
删除元素通常通过查找键然后调用 `erase()` 函数完成,注意需要保证键存在:
```cpp
void removeAlgorithmCategory(const std::string& categoryName) {
auto it = algorithmCategories.find(categoryName);
if (it != algorithmCategories.end()) {
algorithmCategories.erase(it);
} else {
// 错误处理,如果找不到对应键,则抛出异常或返回错误信息
throw std::runtime_error("Category not found");
}
}
```
这两个函数假设 `AbstractEdgeFindCategories` 没有自定义析构函数,因为 `std::shared_ptr` 会自动管理内存。如果你的类有析构需求,记得在 `erase()` 后释放指向的对象。
阅读全文
相关推荐
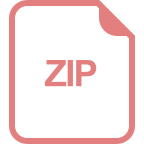
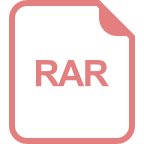




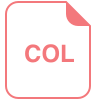
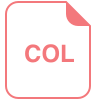
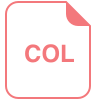

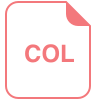

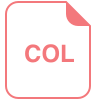
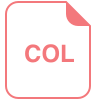
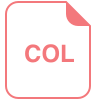
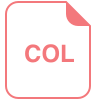
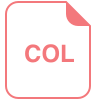
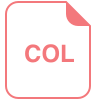
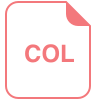