如何用脚本语言(如Python或JavaScript)实现文件的加密操作?
时间: 2024-09-19 18:18:20 浏览: 85
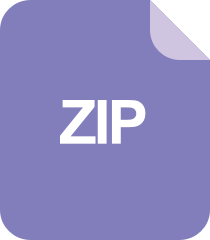
基于JavaScript实现的SM4加密算法设计源码
在Python和JavaScript中,可以使用各种库来实现文件的加密。这里我们以简单的AES(Advanced Encryption Standard)加密为例来说明:
### Python (使用cryptography库)
```python
from cryptography.fernet import Fernet
# 生成密钥
key = Fernet.generate_key()
# 将密钥保存到安全的地方,此处仅示例,实际应用需要妥善处理
with open('secret_key.key', 'wb') as key_file:
key_file.write(key)
def encrypt_file(input_file, output_file):
with open(input_file, 'rb') as file:
data = file.read()
cipher_suite = Fernet(key)
encrypted_data = cipher_suite.encrypt(data)
with open(output_file, 'wb') as encrypted_file:
encrypted_file.write(encrypted_data)
encrypt_file('input.txt', 'output.enc')
```
### JavaScript (使用crypto-js库)
首先,你需要安装`crypto-js`库,可以使用npm:
```bash
npm install crypto-js
```
然后:
```javascript
const CryptoJS = require("crypto-js");
// 加密函数
function encryptFile(inputFile, outputFile) {
let fs = require("fs").promises;
const data = fs.readFile(inputFile, "utf8");
const key = CryptoJS.AES.encrypt(JSON.stringify({ iv: CryptoJS.lib.WordArray.random(16), key: CryptoJS.lib.WordArray.random(CryptoJS.lib.WordArray._length贵Bytes)}), "my-secret-password"); // 使用固定密码替换"my-secret-password"
fs.writeFile(outputFile, JSON.stringify({ ciphertext: key.toString(CryptoJS.enc.Utf8) }), 'utf8');
}
encryptFile('input.txt', 'output.json');
```
注意:上述代码仅用于演示,并非生产环境推荐实践。在实际应用中,应考虑使用更高级的加密技术和合适的密钥管理策略。
阅读全文
相关推荐
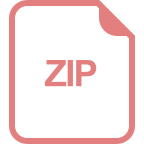
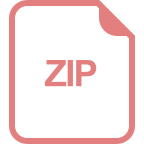















