vue 1. 实现发布评论功能 2. 实现评论列表的展示 3. 使用 tab 栏切换的方式来实现 4. 需要高亮显示当前 tab 栏对应的导航
时间: 2024-11-06 21:16:56 浏览: 25
在Vue.js中实现上述功能,可以按照以下步骤进行:
1. **发布评论功能**:
- 创建一个表单组件(CommentForm.vue),包含输入框、提交按钮等元素。
- 在父组件(如CommentList.vue)里,通过`v-model`绑定表单数据,并监听提交事件,当用户点击提交时,发送请求到后端并更新状态。
```html
<!-- CommentForm.vue -->
<template>
<form @submit.prevent="onSubmit">
<input v-model="commentText" />
<button type="submit">发表</button>
</form>
</template>
<script>
export default {
data() {
return {
commentText: '',
};
},
methods: {
onSubmit() {
this.$emit('comment-added', { text: this.commentText });
this.commentText = '';
},
},
};
</script>
```
2. **评论列表展示**:
- 父组件 CommentList.vue 接收 `comments` 数据,通过`v-for`遍历渲染评论。
```html
<!-- CommentList.vue -->
<template>
<div>
<ul>
<li v-for="comment in comments" :key="comment.id">{{ comment.text }}</li>
</ul>
</div>
</template>
<script>
import CommentForm from './CommentForm.vue';
export default {
components: { CommentForm },
data() {
return {
comments: [],
};
},
//...
</script>
```
3. **Tab栏切换**:
- 使用`vuetify`库的`v-tabs`组件,创建一个`TabComponent.vue`作为内容区域。
- 主组件中引用`v-tabs`,并通过`:active-class`设置高亮样式。
```html
<!-- TabComponent.vue -->
<template>
<v-tabs>
<v-tab key="tab1" to="#tab1-content">评论区</v-tab>
<!-- 添加更多tab选项... -->
</v-tabs>
<router-view></router-view>
</template>
<style scoped>
.active {
/* 设置高亮样式 */
}
</style>
```
4. **高亮当前Tab栏**:
- 使用`v-once`指令防止动态元素影响性能,然后在`TabComponent.vue`中添加事件监听,当路由变化时更新对应tab的激活状态。
```html
<!-- TabComponent.vue (continued) -->
<v-tabs v-once>
<v-tab key="tab1" to="#tab1-content" :class="{ active: $route.hash === '#tab1-content' }">评论区</v-tab>
<!-- ... -->
</v-tabs>
```
阅读全文
相关推荐
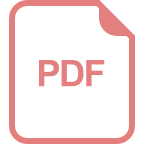
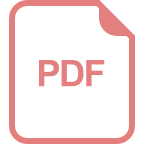
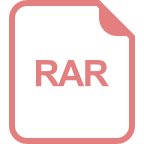
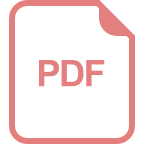
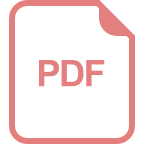
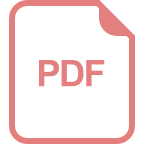
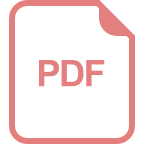
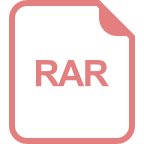
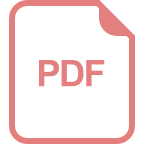
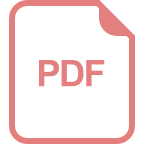
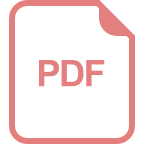
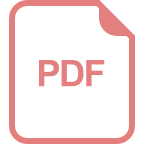
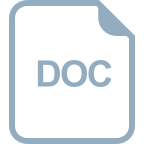
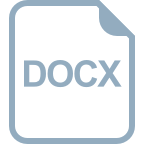
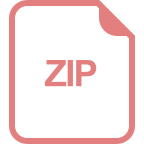