C# winform使用chart每秒钟5000个数据点绘制瀑布图 简单示例代码
时间: 2024-10-22 08:09:48 浏览: 30
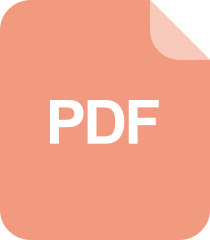
C# WinForm中使用Chart控件绘制曲线图

在C# WinForms应用程序中使用Chart控件绘制瀑布图,并每秒更新5000个数据点,可以通过以下步骤实现。以下是一个简单的示例代码:
1. 创建一个新的WinForms项目。
2. 添加一个Chart控件到你的窗体中。
3. 使用Timer控件来定时更新数据。
下面是具体的代码示例:
```csharp
using System;
using System.Windows.Forms;
using System.Windows.Forms.DataVisualization.Charting;
namespace WaterfallChartExample
{
public partial class Form1 : Form
{
private Random random = new Random();
private int dataPointCount = 0;
private Series series;
public Form1()
{
InitializeComponent();
InitializeChart();
}
private void InitializeChart()
{
chart1.Series.Clear();
series = new Series("Waterfall")
{
ChartType = SeriesChartType.Waterfall,
BorderWidth = 2,
Color = System.Drawing.Color.Blue,
IsValueShownAsLabel = true
};
chart1.Series.Add(series);
// Set up the chart area
ChartArea chartArea = new ChartArea();
chart1.ChartAreas.Add(chartArea);
// Set up the legend
Legend legend = new Legend();
legend.Name = "Legend";
chart1.Legends.Add(legend);
// Set up the timer to update the chart every second
Timer timer = new Timer();
timer.Interval = 1000; // 1 second
timer.Tick += Timer_Tick;
timer.Start();
}
private void Timer_Tick(object sender, EventArgs e)
{
UpdateChart();
}
private void UpdateChart()
{
for (int i = 0; i < 5000; i++)
{
double value = random.NextDouble() * 10 - 5; // Generate random values between -5 and 5
series.Points.AddXY(dataPointCount++, value);
}
}
}
}
```
### 解释:
1. **初始化Chart控件**:在`InitializeChart`方法中,我们创建了一个`Series`对象,并将其类型设置为`SeriesChartType.Waterfall`。我们还设置了图表区域和图例。
2. **定时器**:使用`Timer`控件每秒触发一次`Timer_Tick`事件,以更新图表的数据。
3. **更新图表**:在`UpdateChart`方法中,我们生成了5000个随机数据点,并将它们添加到`Series`中。这些数据点将显示为瀑布图中的柱状条。
### 注意事项:
- 确保你已经在项目中引用了`System.Windows.Forms.DataVisualization`命名空间。
- 你可能需要调整图表的样式和属性以满足你的需求。
- 由于每秒更新5000个数据点,这可能会导致性能问题,特别是在低配置的机器上。如果遇到性能问题,可以考虑优化数据更新逻辑或减少数据点的数量。
阅读全文
相关推荐
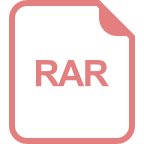
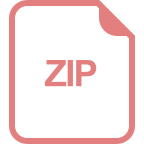
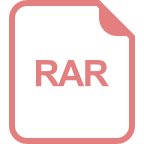
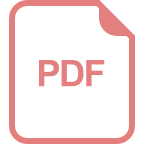
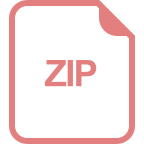
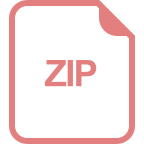
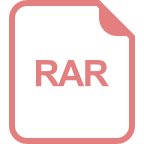
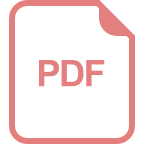
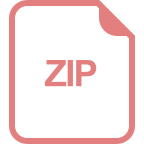
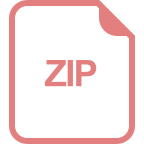
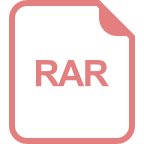
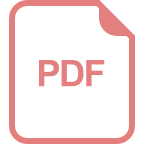
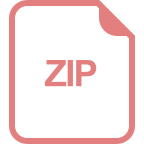
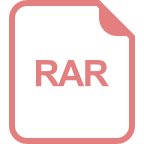
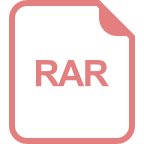
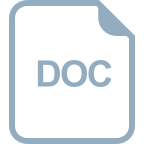