c语言有5个学生,每个学生的数据包括学号、姓名、3门课的成绩。从键盘输入5个学生数据,要求输出3门课总平均成绩,以及平均成绩最高分的学生的数据(包括学号、姓名、3门课的成绩、平均分)
时间: 2023-12-16 17:28:31 浏览: 38
```c
#include <stdio.h>
#include <string.h>
struct Student {
char id[10];
char name[20];
float score[3];
float avg;
};
int main() {
struct Student stu[5];
float sum[3] = {0};
float maxAvg = 0;
int maxIndex = 0;
// 输入学生数据
for (int i = 0; i < 5; i++) {
printf("请输入第%d个学生的学号:", i+1);
scanf("%s", stu[i].id);
printf("请输入第%d个学生的姓名:", i+1);
scanf("%s", stu[i].name);
printf("请输入第%d个学生的3门课成绩:", i+1);
for (int j = 0; j < 3; j++) {
scanf("%f", &stu[i].score[j]);
sum[j] += stu[i].score[j];
}
stu[i].avg = (stu[i].score[0] + stu[i].score[1] + stu[i].score[2]) / 3;
if (stu[i].avg > maxAvg) {
maxAvg = stu[i].avg;
maxIndex = i;
}
}
// 输出3门课总平均成绩
printf("3门课总平均成绩为:%.2f %.2f %.2f\n", sum[0]/5, sum[1]/5, sum[2]/5);
// 输出平均成绩最高分的学生数据
printf("平均成绩最高分的学生数据为:\n");
printf("学号:%s\n", stu[maxIndex].id);
printf("姓名:%s\n", stu[maxIndex].name);
printf("3门课成绩:%.2f %.2f %.2f\n", stu[maxIndex].score[0], stu[maxIndex].score[1], stu[maxIndex].score[2]);
printf("平均分:%.2f\n", stu[maxIndex].avg);
return 0;
}
```
相关推荐
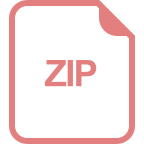
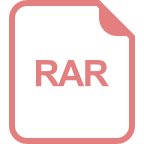
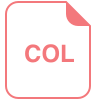
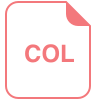
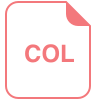
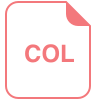
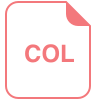









