xgboost模拟预测代码
时间: 2023-08-13 17:03:27 浏览: 39
当使用XGBoost进行模拟预测时,你需要先导入必要的库并加载训练好的模型。然后,你可以使用模型来预测新的数据样本。下面是一个简单的示例代码:
```python
import xgboost as xgb
import pandas as pd
# 加载训练好的模型
model = xgb.Booster(model_file='path_to_model_file')
# 加载新的数据样本
new_data = pd.read_csv('path_to_new_data')
# 进行预测
preds = model.predict(xgb.DMatrix(new_data))
# 打印预测结果
print(preds)
```
在这个示例中,你需要将`path_to_model_file`替换为你训练好的模型文件的路径,将`path_to_new_data`替换为包含新数据样本的CSV文件的路径。预测结果将会被打印出来。
请确保在运行代码之前已经安装了XGBoost库,并且已经训练好了模型并保存为文件。
相关问题
xgboost回归预测代码
xgboost是一种强大的机器学习算法,常用于进行回归预测。以下是一个简单的xgboost回归预测代码示例:
首先,需要导入必要的库:
```
import xgboost as xgb
import numpy as np
from sklearn.metrics import mean_squared_error
from sklearn.model_selection import train_test_split
```
然后,加载数据集并进行数据准备:
```
# 加载数据集
dataset = np.loadtxt('data.csv', delimiter=',')
# 划分特征和标签
X = dataset[:, 0:4]
y = dataset[:, 4]
```
接下来,将数据集划分为训练集和测试集:
```
# 将数据集划分为训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=0)
```
然后,配置xgboost回归模型的参数:
```
# 配置模型参数
params = {
'max_depth': 3,
'eta': 0.1,
'objective': 'reg:squarederror',
'eval_metric': 'rmse'
}
```
接下来,对训练集进行训练并预测测试集:
```
# 将数据转换为DMatrix格式
dtrain = xgb.DMatrix(X_train, label=y_train)
dtest = xgb.DMatrix(X_test)
# 训练模型
model = xgb.train(params, dtrain)
# 预测测试集
y_pred = model.predict(dtest)
```
最后,计算模型的均方根误差(RMSE)来评估回归预测的准确性:
```
# 计算均方根误差
rmse = np.sqrt(mean_squared_error(y_test, y_pred))
print("RMSE:", rmse)
```
这就是一个简单的xgboost回归预测代码的示例。根据实际需求,你可以根据数据集和模型的参数进行相应的调整和优化。
xgboost预测matlab代码
我们可以使用SSA-XGBoost算法来进行时间序列预测,需要进行一些参数调优。首先,在选择学习速率时,一般情况下取0.1。然后,需要确定对应于该学习速率的理想决策树数量。可以使用XGBoost中的交叉验证函数“cv”来获得理想的决策树数量。接下来,我们需要对决策树的特定参数进行调优,例如最大深度(max_depth)、最小权重(min_child_weight)、gamma值、样本子采样率(subsample)和列采样率(colsample_bytree)。另外,还需要进行正则化参数(lambda和alpha)的调优,以减少模型复杂度并提高性能。最后,可以降低学习速率并确定理想参数。以下是一个简化版本的XGBoost预测Matlab代码示例:
```matlab
% 导入数据
data = load('your_data.mat');
X_train = data.X_train;
y_train = data.y_train;
X_test = data.X_test;
% 设置参数
params = struct();
params.learning_rate = 0.1;
params.n_estimators = 100;
params.max_depth = 3;
params.min_child_weight = 1;
params.gamma = 0;
params.subsample = 1;
params.colsample_bytree = 1;
params.reg_alpha = 0;
params.reg_lambda = 1;
% 训练模型
model = xgboostTrain(X_train, y_train, params);
% 进行预测
y_pred = xgboostPredict(model, X_test);
```
在这个示例代码中,我们首先导入数据,然后设置了一些参数,包括学习速率、决策树数量、最大深度、最小权重、gamma值、样本子采样率、列采样率、正则化参数等。接下来,使用训练数据训练模型,并使用测试数据进行预测。最终得到的预测结果保存在变量y_pred中。
请注意,这只是一个简化版本的代码示例,实际应用中可能需要根据具体情况进行更详细的参数调优和模型训练过程。<span class="em">1</span><span class="em">2</span><span class="em">3</span><span class="em">4</span>
相关推荐
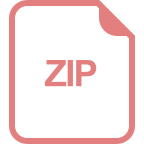












