java 从fileNet中高效下载文件
时间: 2024-10-20 22:04:02 浏览: 21
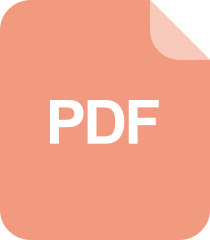
F005_1 Filenet CE JAVA API.pdf

在Java中,从FileNet高效地下载文件通常涉及使用FTP (File Transfer Protocol) 或HTTP(S)库,如Apache Commons Net或Java内置的URLConnection。以下是基本步骤:
1. 导入所需的库:
```java
import org.apache.commons.net.ftp.FTPClient;
import java.io.FileOutputStream;
import java.io.InputStream;
import java.net.URL;
```
2. 对于FTP:
```java
FTPClient ftp = new FTPClient();
try {
// 连接到FTP服务器
ftp.connect("ftp.example.com", 21);
ftp.login("username", "password");
// 选择目录
ftp.changeWorkingDirectory("/path/to/download/folder");
// 打开并下载文件
InputStream fis = ftp.retrieveFileStream("filename.zip");
FileOutputStream fos = new FileOutputStream("local_file_path.zip");
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = fis.read(buffer)) != -1) {
fos.write(buffer, 0, bytesRead);
}
fos.close();
fis.close();
// 关闭连接
ftp.logout();
ftp.disconnect();
} catch (Exception e) {
e.printStackTrace();
}
```
3. 对于HTTP(S):
```java
URL url = new URL("https://example.com/file.zip");
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
try {
connection.setRequestMethod("GET");
connection.connect();
// 获取输入流
InputStream in = connection.getInputStream();
FileOutputStream fos = new FileOutputStream("local_file_path.zip");
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = in.read(buffer)) != -1) {
fos.write(buffer, 0, bytesRead);
}
fos.close();
in.close();
connection.disconnect();
} catch (IOException e) {
e.printStackTrace();
}
```
阅读全文
相关推荐
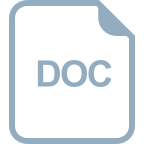
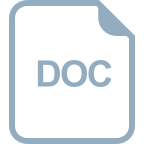
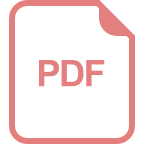
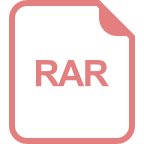
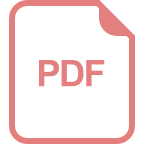
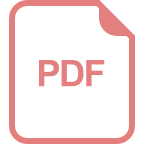
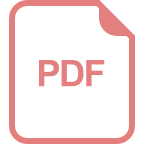
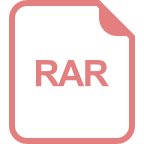
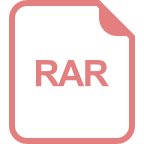
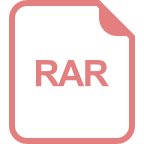
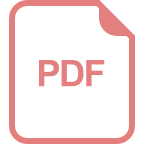
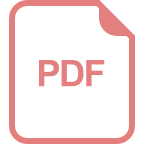
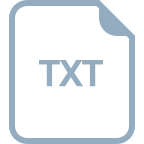
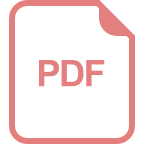
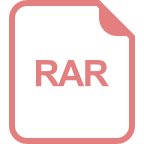
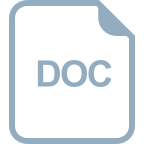
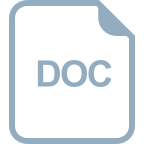
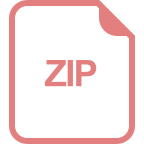
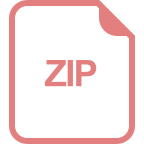