vue excel 预览
时间: 2023-07-17 22:07:08 浏览: 246
要在Vue应用中预览Excel文件,你可以使用一些现有的库和插件来实现。以下是一种常见的方法,使用`xlsx`库来读取和解析Excel文件,并使用`vue-good-table`库来显示Excel数据。
1. 首先,安装所需的库:
```bash
npm install xlsx vue-good-table
```
2. 创建一个Vue组件,并导入所需的库:
```vue
<template>
<div>
<vue-good-table :columns="columns" :rows="rows" />
</div>
</template>
<script>
import { read, utils } from 'xlsx';
import VueGoodTablePlugin from 'vue-good-table';
import 'vue-good-table/dist/vue-good-table.css';
export default {
components: {
VueGoodTable: VueGoodTablePlugin,
},
data() {
return {
columns: [],
rows: [],
};
},
mounted() {
this.loadExcelFile();
},
methods: {
loadExcelFile() {
const file = 'path/to/your/excel/file.xlsx';
const reader = new FileReader();
reader.onload = (e) => {
const data = new Uint8Array(e.target.result);
const workbook = read(data, { type: 'array' });
const worksheet = workbook.Sheets[workbook.SheetNames[0]];
const jsonData = utils.sheet_to_json(worksheet, { header: 1 });
// 解析列头
this.columns = jsonData[0].map((header) => ({
label: header,
field: header,
}));
// 解析数据行
this.rows = jsonData.slice(1).map((row) =>
row.reduce((acc, value, index) => {
acc[this.columns[index].field] = value;
return acc;
}, {})
);
};
reader.readAsArrayBuffer(file);
},
},
};
</script>
```
在上面的代码中,你需要将`path/to/your/excel/file.xlsx`替换为实际Excel文件的路径。组件将读取Excel文件并解析为列和行数据,然后使用`vue-good-table`来呈现表格。
请注意,该示例仅提供了一种基本实现方法。你可以根据自己的需求进行修改和调整。
希望这可以帮助到你!如果你有其他问题,请随时提问。
阅读全文
相关推荐
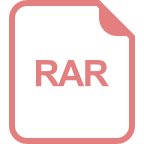
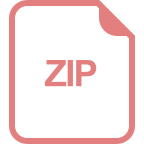


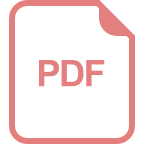











