计算倒计时天数计算公式C语言
时间: 2024-08-30 12:00:31 浏览: 112
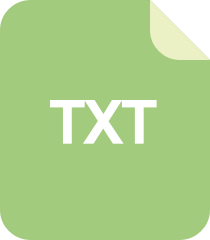
C语言实现天数倒计时功能
在C语言中,计算倒计时天数的基本思路是从当前日期开始,减去给定的结束日期。这里我们可以使用`<time.h>`库中的时间处理函数。假设我们有一个结束日期(例如整型结构体表示年、月、日),你可以使用以下步骤:
```c
#include <stdio.h>
#include <time.h>
// 定义一个结构体表示日期
typedef struct {
int year;
int month;
int day;
} Date;
// 函数计算两个日期之间的天数差
int days_between(Date end_date, Date current_date) {
time_t end_time = mktime(&(end_date.year * 10000 + end_date.month * 100 + end_date.day));
time_t current_time = mktime(&(current_date.year * 10000 + current_date.month * 100 + current_date.day));
if (end_time > current_time) {
// 如果结束日期在将来,则取负值,因为mtime返回的是距离1970年1月1日的时间差
return -difftime(end_time, current_time);
} else {
return difftime(end_time, current_time);
}
}
int main() {
// 示例:假设目标日期是2023年12月31日
Date target = {2023, 12, 31};
Date today;
// 获取当前日期
time_t now = time(0);
struct tm* t = localtime(&now);
today.year = t->tm_year + 1900;
today.month = t->tm_mon + 1;
today.day = t->tm_mday;
int countdown = days_between(target, today);
printf("倒计时天数: %d 天\n", countdown);
return 0;
}
```
阅读全文
相关推荐
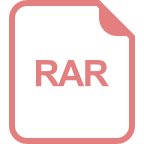
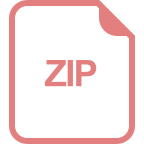
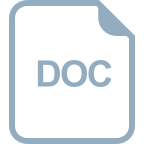
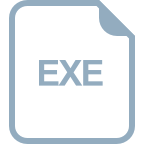
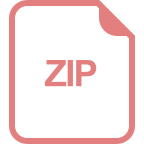
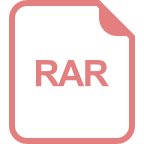
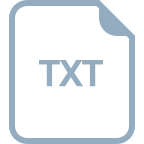
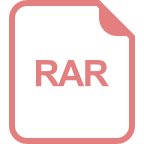
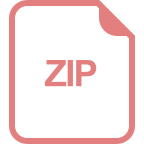
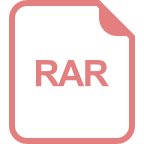
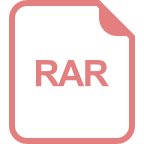
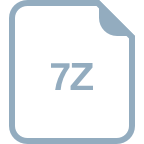
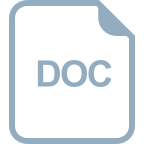