用c语言编写一份完整的最佳适应算法
时间: 2024-11-22 14:38:25 浏览: 1
在C语言中,编写一个简单的最佳适应(Best Fit)算法通常用于内存管理,特别是动态内存分配中,当需要为一组大小未知的对象寻找最小连续内存块时。下面是一个基本的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#define CHUNK_SIZE 10 // 内存块大小
#define MAX_CHUNKS 100 // 最大可以分割的内存块数
// 结构体表示内存块
typedef struct {
void* start; // 块起始地址
size_t size; // 块大小
} MemoryChunk;
// 比较函数,用于比较两个内存块
int compare(const void *a, const void *b) {
return (*(MemoryChunk*)a).size - (*(MemoryChunk*)b).size;
}
void best_fit(int n, MemoryChunk* chunks) {
qsort(chunks, n, sizeof(MemoryChunk), compare); // 对内存块进行排序
for (int i = 0; i < n; ++i) {
void* smallest = chunks[0].start; // 找到当前最小的
int smallest_index = 0;
// 遍历已排序的块,直到找到足够大的
for (int j = 1; j < n; ++j) {
if (*(chunks[j].start) + chunks[j].size <= smallest + CHUNK_SIZE) { // 如果余量大于等于新块大小
smallest = chunks[j].start;
smallest_index = j;
}
}
printf("Assigning to block %d of size %zu at address %p\n", smallest_index, CHUNK_SIZE, smallest);
// 这里只是打印信息,实际应用中你需要分配内存并记录对应索引
}
}
int main() {
int n; // 输入对象的数量
printf("Enter the number of objects: ");
scanf("%d", &n);
MemoryChunk chunks[MAX_CHUNKS];
// 初始化内存块列表...
best_fit(n, chunks);
return 0;
}
```
阅读全文
相关推荐
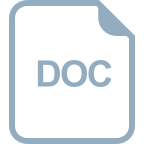
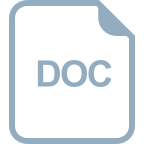
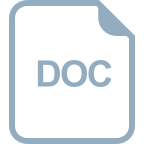
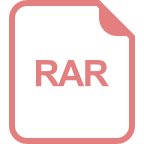
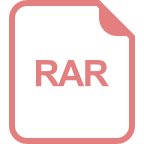
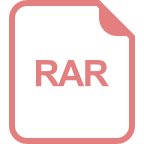
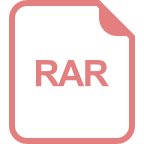
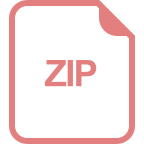
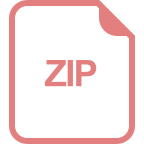
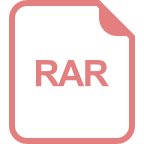
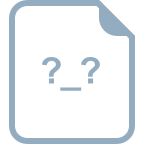
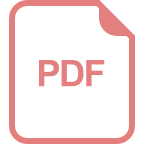
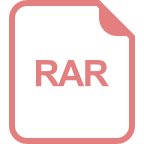
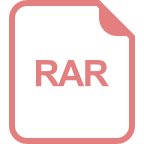
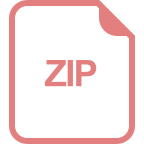
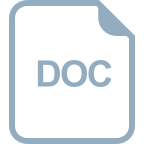
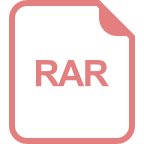
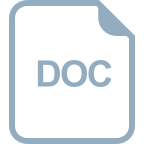
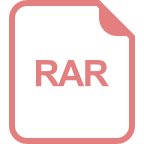