c++写一个简易加密解密代码
时间: 2024-10-14 11:02:47 浏览: 7
你可以使用C++语言编写一个简易的加密解密代码。下面是一个简单的示例:
1. 加密算法
你可以使用一些简单的算法来加密你的数据,例如替换、移位、异或等。下面是一个简单的替换算法的示例:
```c++
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
int main()
{
string key;
ifstream keyFile("key.txt");
getline(keyFile, key);
keyFile.close();
string sourceFile, targetFile;
cout << "Enter source file path: ";
getline(cin, sourceFile);
cout << "Enter target file path: ";
getline(cin, targetFile);
ifstream source(sourceFile);
ofstream target(targetFile);
char c;
while (source.get(c))
{
if (c >= 'a' && c <= 'z')
{
c = key[c - 'a'];
}
else if (c >= 'A' && c <= 'Z')
{
c = key[c - 'A'] - 'a' + 'A';
}
target << c;
}
source.close();
target.close();
cout << "Encryption completed." << endl;
return 0;
}
```
2. 解密算法
解密算法与加密算法相反,可以使用相同的算法来解密数据。下面是一个简单的解密算法的示例:
```c++
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
int main()
{
string key;
ifstream keyFile("key.txt");
getline(keyFile, key);
keyFile.close();
string sourceFile, targetFile;
cout << "Enter source file path: ";
getline(cin, sourceFile);
cout << "Enter target file path: ";
getline(cin, targetFile);
ifstream source(sourceFile);
ofstream target(targetFile);
char c;
while (source.get(c))
{
if (c >= 'a' && c <= 'z')
{
c = 'a' + key.find(c);
}
else if (c >= 'A' && c <= 'Z')
{
c = 'A' + key.find(c - 'A' + 'a');
}
target << c;
}
source.close();
target.close();
cout << "Decryption completed." << endl;
return 0;
}
```
阅读全文
相关推荐
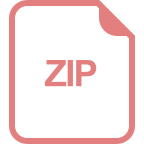
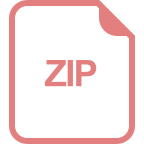
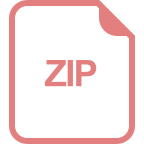















