编写程序,完成栈和队列的基本操作。要求使用链栈与顺序队列实现,数据域自定,但不能少于两项属性。并能够演示各项功能,用C语言完成
时间: 2024-11-06 19:19:49 浏览: 32
在C语言中,我们可以分别使用链表结构(链栈)和数组(顺序队列)来实现栈和队列的基本操作。这里是一个简单的示例:
首先,我们定义两个结构体,`StackNode` 和 `QueueNode` 分别代表链栈节点和队列节点,包含数据域以及指向下一个元素的指针。
```c
typedef struct StackNode {
int data;
struct StackNode* next; // 链栈节点的链接指针
} StackNode;
typedef struct QueueNode {
int data;
struct QueueNode* front; // 顺序队列的头指针
struct QueueNode* rear; // 尾指针
} QueueNode;
```
然后,我们创建链栈和顺序队列的数据结构,并实现基本操作:
```c
// 初始化空栈和队列
StackNode* stack_init() { return NULL; }
QueueNode* queue_init() { QueueNode* front = rear = NULL; return front; }
// 入栈(链栈)
void push(StackNode** top, int value) {
StackNode* newNode = (StackNode*)malloc(sizeof(StackNode));
newNode->data = value;
newNode->next = *top;
*top = newNode;
}
// 出栈(链栈)
int pop(StackNode** top) {
if (*top == NULL) return -1; // 空栈处理
int val = (*top)->data;
StackNode* temp = *top;
*top = (*top)->next;
free(temp);
return val;
}
// 入队(顺序队列)
void enqueue(QueueNode** front, QueueNode** rear, int value) {
QueueNode* newNode = (QueueNode*)malloc(sizeof(QueueNode));
newNode->data = value;
newNode->rear = *rear;
if (*front != NULL) newNode->front = *front;
else newNode->front = newNode; // 如果队列为空,则front和rear同时指向新节点
*rear = newNode;
}
// 出队(顺序队列)
int dequeue(QueueNode** front) {
if (*front == NULL) return -1; // 空队列处理
int val = (*front)->data;
QueueNode* temp = *front;
*front = (*front)->front;
if (*front == NULL) *rear = NULL; // 队列变空时更新rear
free(temp);
return val;
}
```
现在,你可以通过调用上述函数来进行栈和队列的操作,并观察它们的行为。例如,你可以先初始化栈和队列,然后依次尝试入栈、出栈、入队、出队等操作,验证其有效性。
阅读全文
相关推荐



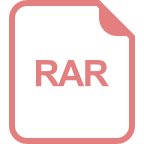


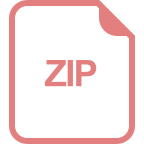






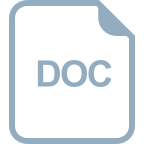



