用c语言编写一个函数,计算数组中出现次数超过n/3的元素(n是数组的长度)。可以使用哈希表或摩尔投票法。
时间: 2024-12-14 11:16:57 浏览: 8
在C语言中,我们可以使用哈希表(通过结构体数组和链表)或者摩尔投票法(位运算)来解决这个问题。这里我将展示如何使用哈希表的方法:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_HASH_SIZE 100
typedef struct {
int element;
int count;
} HashEntry;
void add_to_hash(HashEntry* hash_table, int element) {
for (int i = 0; i < MAX_HASH_SIZE; ++i) {
if (hash_table[i].element == element) {
hash_table[i].count++;
return;
}
}
// 如果不存在该元素,添加新的项
HashEntry new_entry = {element, 1};
hash_table[MAX_HASH_SIZE - 1] = new_entry;
}
int find_majority(int arr[], int n, HashEntry* hash_table) {
int majority_count = n / 3 + 1;
for (int i = 0; i < n; ++i) {
add_to_hash(hash_table, arr[i]);
if (hash_table[arr[i]].count > majority_count) {
majority_count = hash_table[arr[i]].count;
majority_element = arr[i];
}
}
return majority_element;
}
int main() {
int arr[] = {1, 2, 2, 3, 3, 3, 4, 5, 5, 5};
int n = sizeof(arr) / sizeof(arr[0]);
HashEntry hash_table[MAX_HASH_SIZE];
int majority_element = find_majority(arr, n, hash_table);
printf("The element that appears more than n/3 times is: %d\n", majority_element);
return 0;
}
```
在这个程序中,我们首先创建了一个大小为MAX_HASH_SIZE的哈希表。`add_to_hash`函数用于更新每个元素及其计数。`find_majority`函数遍历数组,不断更新哈希表,并寻找出现次数超过n/3的元素。
注意,这个实现假设输入数组有整数元素,并且元素数量不大于哈希表的最大容量。如果你需要处理更复杂的情况,可能需要调整代码或者选择其他数据结构。
阅读全文
相关推荐









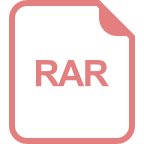








