多种方式实现两个开关控制led灯 c语言程序
时间: 2024-09-15 15:10:47 浏览: 52
在C语言中,你可以通过结构体、函数指针或者联合体来实现两个开关分别控制LED灯的功能。这里简单给出几种方法:
**方法一:使用结构体**
```c
#include <stdio.h>
typedef struct {
int ledState;
void (*switchAction)(void);
} LedControl;
void switchOn() {
printf("Switch 1 is on, turning LED on.\n");
}
void switchOff() {
printf("Switch 1 is off, turning LED off.\n");
}
int main() {
LedControl light = {0, NULL}; // 初始状态关闭
light.switchAction = switchOn; // 设置初始切换动作
while (1) {
if (getchar() == '1') { // 用户输入1开启,2关闭
light.switchAction = switchOn;
} else if (getchar() == '2') {
light.switchAction = switchOff;
}
light.switchAction(); // 执行当前开关动作
}
return 0;
}
```
**方法二:使用函数指针**
```c
#include <stdio.h>
void switchLedOn() {
printf("Switch 2 is on, turning LED on.\n");
}
void switchLedOff() {
printf("Switch 2 is off, turning LED off.\n");
}
int main() {
void (*ledActions[])(void) = {NULL, &switchLedOn, &switchLedOff};
int currentActionIndex = 0;
while (1) {
char input;
scanf("%d", &input);
if (input == 1) {
ledActions[currentActionIndex] = switchLedOff;
} else if (input == 2) {
ledActions[currentActionIndex] = switchLedOn;
}
ledActions[currentActionIndex](); // 执行当前操作
currentActionIndex = (currentActionIndex + 1) % 3; // 循环切换
}
return 0;
}
```
**方法三:使用联合体+位运算**
```c
#include <stdio.h>
union LedState {
unsigned char byte;
struct {
unsigned char switch1 : 1;
unsigned char switch2 : 1;
unsigned char unused : 6;
} bits;
};
void setLed(int switchNumber, int state) {
union LedState led;
led.bits.switch1 = switchNumber == 1 ? state : 0;
led.byte |= (state << (switchNumber * 2)); // 高两位对应开关1和2
printf("Switch %d: %s\n", switchNumber, state ? "on" : "off");
}
int main() {
while (1) {
int switchInput;
scanf("%d", &switchInput);
setLed(switchInput, 1); // 开启
setLed(2 - switchInput, 0); // 关闭另一个
}
return 0;
}
```
相关推荐
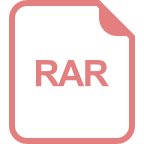
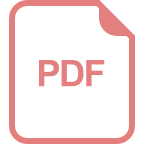
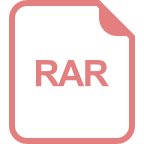














