service层如何调用mybatis.mapper.xml文件中的多个sql语句功能,并且在controller层使用查询接口调用全部功能
时间: 2024-11-15 10:20:45 浏览: 41
在Spring Boot项目中,Service层通常不会直接操作`mybatis.mapper.xml`文件,而是通过`Mapper`接口和`SqlSession`来调用SQL。首先,你需要创建一个`Mapper`接口,这个接口会对应`mapper.xml`中的映射:
```java
public interface MyMapper {
List<User> getAllUsers(); // 映射到xml中的第一个SQL
int deleteUser(int id); // 映射到xml中的第二个SQL
}
```
然后,在`MyService`服务类中,你可以注入对应的`Mapper`并使用`SqlSession`执行SQL:
```java
@Service
public class MyServiceImpl implements MyService {
@Autowired
private MyMapper myMapper;
public void processData() {
// 调用第一个SQL获取所有用户
List<User> allUsers = myMapper.getAllUsers();
// 调用第二个SQL删除某个用户
int deletedId = myMapper.deleteUser(1);
// 其他业务处理...
}
}
```
在Controller层,你可以像下面这样通过`@Autowired`注入Service实例并调用其方法:
```java
@RestController
@RequestMapping("/api")
public class UserController {
@Autowired
private MyService myService;
@GetMapping("/users")
public ResponseEntity<List<User>> getUsers() {
List<User> users = myService.processData();
// 返回数据给前端
return ResponseEntity.ok(users);
}
// 其他HTTP映射方法...
}
```
每个请求到达`/api/users`路径时,`getUsers`方法会被调用,进而执行Service层的`processData`方法,实现了对两个SQL的并发调用。
阅读全文
相关推荐
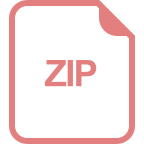
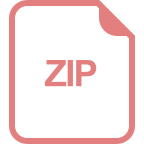
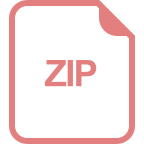
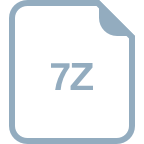
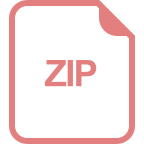
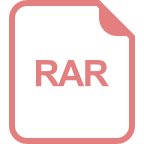
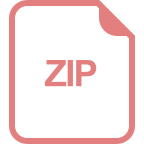
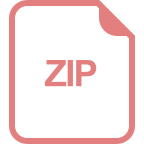
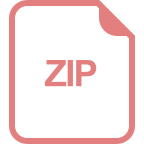
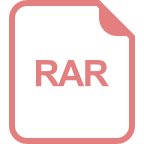
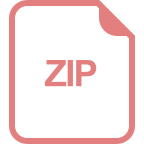
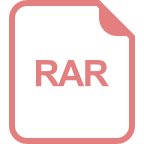
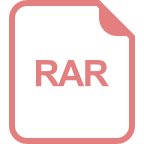
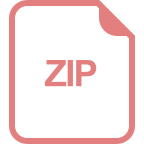
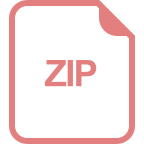
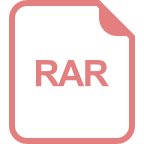
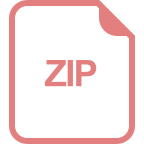