使用反射给某个方法添加注解
时间: 2024-03-01 22:16:29 浏览: 196
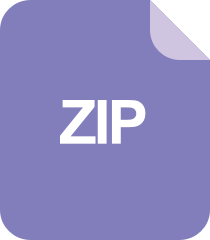
java 通过反射获取类上注解,方法上注解,注解里的值及方法参数
要给某个方法添加注解,可以使用反射来实现。下面是一个示例代码:
```java
import java.lang.annotation.Annotation;
import java.lang.reflect.Method;
public class AnnotationExample {
public static void main(String[] args) throws NoSuchMethodException {
// 获取要添加注解的方法
Method method = MyClass.class.getMethod("myMethod");
// 获取注解类的实例
MyAnnotation annotation = method.getAnnotation(MyAnnotation.class);
// 如果方法已经有注解,则输出注解的值
if (annotation != null) {
System.out.println("MyAnnotation value: " + annotation.value());
}
// 动态添加注解
if (annotation == null) {
// 获取注解类
Class<MyAnnotation> annotationClass = MyAnnotation.class;
// 创建一个注解实例
MyAnnotation newAnnotation = annotationClass.newInstance();
// 设置注解的值
newAnnotation.setValue("New Value");
// 获取方法的注解数组
Annotation[] annotations = method.getDeclaredAnnotations();
// 创建一个新的注解数组,长度比原数组多1
Annotation[] newAnnotations = new Annotation[annotations.length + 1];
// 将原数组的元素复制到新数组中
System.arraycopy(annotations, 0, newAnnotations, 0, annotations.length);
// 将新注解添加到新数组的最后一个位置
newAnnotations[newAnnotations.length - 1] = newAnnotation;
// 使用反射设置方法的注解数组
Method annotationsMethod = Method.class.getDeclaredMethod("declaredAnnotations");
annotationsMethod.setAccessible(true);
annotationsMethod.invoke(method)[0] = newAnnotations;
}
}
}
// 自定义注解
@interface MyAnnotation {
String value() default "";
}
// 被注解的类
class MyClass {
@MyAnnotation("Old Value")
public void myMethod() {
System.out.println("MyMethod");
}
}
```
上述代码中,首先通过反射获取要添加注解的方法。然后,判断方法是否已经有注解,如果有则输出注解的值。如果方法没有注解,则使用反射动态添加注解。具体实现是通过创建一个新的注解实例,并设置注解的值,然后将新注解添加到方法的注解数组中。最后使用反射设置方法的注解数组。这样就成功给方法添加了注解。
阅读全文
相关推荐
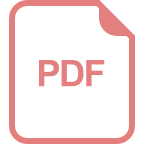
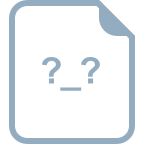
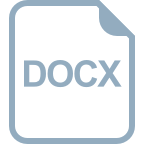
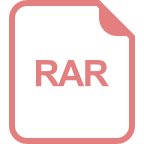
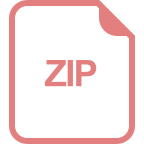
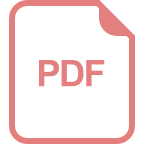
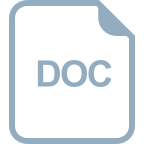
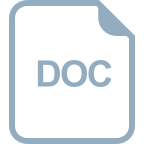
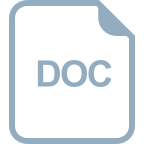
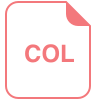
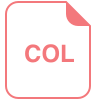
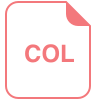
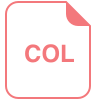
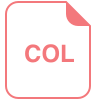