springboot 添加mybatis拦截器,给所有表名添前缀
时间: 2024-09-12 19:14:44 浏览: 64
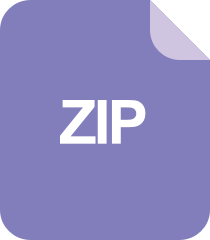
SpringBoot整合Mybatis完整详细版含注册、登录、拦截器配置
在Spring Boot项目中集成MyBatis并添加全局拦截器,以便为所有表名添加前缀,通常需要以下几个步骤:
1. 首先,在Spring Boot项目的`application.properties`或`application.yml`配置文件中添加对MyBatis的依赖,如果尚未启用,可以添加类似以下内容:
```properties
mybatis.type-aliases-package=com.example.mapper
```
2. 创建一个自定义的全局SQL映射器(GlobalMapper.xml),这将在所有其他映射器之前加载,可以在`src/main/resources/Mapper`目录下创建:
```xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.example.globalMapper">
<!-- 全局前缀设置 -->
<resultMap id="baseResultMap" type="your.BaseEntity">
<id column="id" property="id" jdbcType="INTEGER"/>
<result column="prefix_table_name_column" property="tableNameColumn" jdbcType="VARCHAR"/>
<!-- 其他字段... -->
</resultMap>
</mapper>
```
3. 定义一个全局拦截器(GlobalInterceptor.java),这个拦截器将在每个SQL查询执行之前应用前缀:
```java
@Component
public class GlobalInterceptor implements Interceptor {
private final String tableNamePrefix;
public GlobalInterceptor(String tableNamePrefix) {
this.tableNamePrefix = tableNamePrefix;
}
@Override
public Object intercept(Invocation invocation) throws Throwable {
Executor executor = (Executor) invocation.getTarget();
BoundSql boundSql = (BoundSql) invocation.getArgs()[0];
boundSql.setTable(tableNamePrefix + boundSql.getTable());
return executor.query(boundSql);
}
@Override
public Object plugin(Object target) {
if (target instanceof Executor) {
return Plugin.wrap(target, this);
}
return target;
}
@Override
public void setProperties(Properties properties) {}
}
```
4. 最后,在MyBatis的配置类(如`MybatisConfig.java`)中注入自定义的全局拦截器,并注册它:
```java
@Configuration
public class MybatisConfig extends SqlSessionFactoryBean {
@Autowired
private GlobalInterceptor globalInterceptor;
@PostConstruct
public void init() {
SqlSessionBuilder builder = new SqlSessionFactoryBuilder().build(this);
builder.interceptors().add(globalInterceptor);
this.sqlSessionFactory = builder.build();
}
}
```
阅读全文
相关推荐
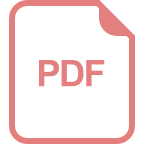
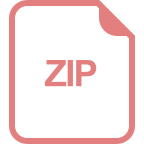
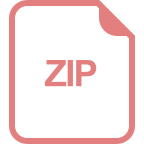
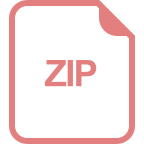
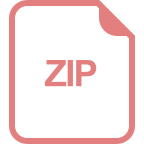
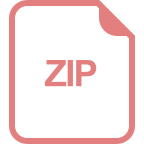
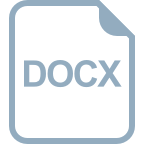
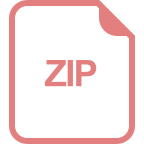
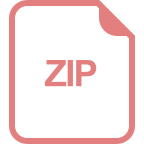
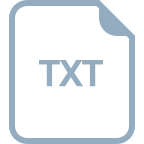
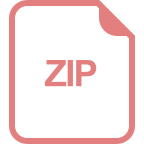
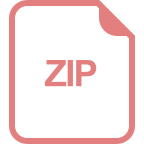
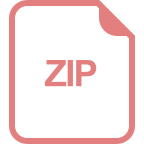
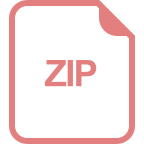
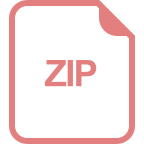
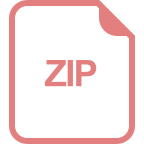