C中的volatile作用
时间: 2024-02-22 13:52:15 浏览: 23
C语言中的volatile关键字用于告诉编译器,该变量的值可能会在程序执行期间被意外地改变,因此编译器不应该对该变量进行优化。volatile关键字通常用于以下情况:
1.多线程或多进程环境中,某个变量被多个线程或进程共享,且可能被其他线程或进程修改。
2.中断服务程序中,某个变量被中断程序和主程序共享,且可能被中断程序修改。
3.某个变量的值可能被外部设备或其他程序修改。
以下是几个使用volatile关键字的例子:
1.在多线程环境中,使用volatile关键字来确保变量的可见性和一致性:
```c
volatile int count = 0;
void* thread_func(void* arg) {
while (count < 100) {
// do something
}
return NULL;
}
int main() {
pthread_t tid;
pthread_create(&tid, NULL, thread_func, NULL);
while (count < 100) {
count++;
// do something
}
pthread_join(tid, NULL);
return 0;
}
```
2.在中断服务程序中,使用volatile关键字来确保变量的可见性和一致性:
```c
volatile int flag = 0;
void isr() {
flag = 1;
}
int main() {
while (1) {
if (flag) {
// do something
flag = 0;
}
}
return 0;
}
```
3.在使用指针时,使用volatile关键字来确保指针所指向的值不被优化掉:
```c
volatile int* ptr = (int*)0x1000;
int value = *ptr;
```
相关推荐
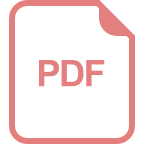














