用python绘制一台3d的电脑
时间: 2024-11-27 10:22:51 浏览: 4
在Python中,我们可以使用matplotlib库结合mplot3d工具包来绘制三维图形,比如简单的3D电脑模型并不直接内置,但我们可以通过创建一些几何形状组合来模拟。这里是一个基础示例,展示如何创建一个简单的立方体代表电脑主机:
```python
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d.art3d import Poly3DCollection
# 创建电脑主机的立方体
def create_host(x, y, z):
vertices = [(x, y, z), (x, y + 1, z), (x + 1, y + 1, z), (x + 1, y, z)]
faces = [[0, 1, 2, 3]] # 四面墙
host = Poly3DCollection([vertices], alpha=0.5, color='gray')
return host
# 创建显示器的长方体
def create_monitor(x, y, z):
vertices = [
(x, y - 0.5, z),
(x + 1, y - 0.5, z),
(x + 1, y + 0.5, z),
(x, y + 0.5, z)
]
faces = [[0, 1, 2, 3]]
monitor = Poly3DCollection([vertices], alpha=0.8, color='blue')
return monitor
# 绘制
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
host = create_host(0, 0, 0) # 主机位置
monitor = create_monitor(-1, 0, 0) # 显示器位置,稍微偏移
ax.add_collection3d(host)
ax.add_collection3d(monitor)
ax.set_xlim([-2, 2])
ax.set_ylim([-1, 2])
ax.set_zlim([-1, 1])
plt.show()
阅读全文
相关推荐
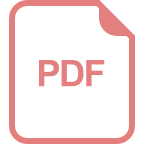
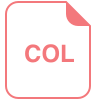

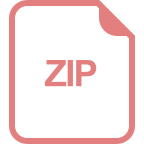
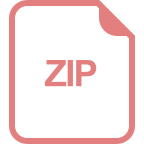
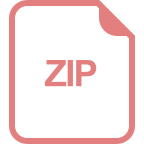
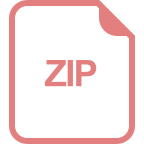
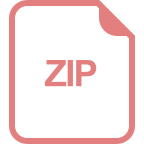
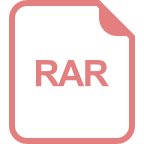
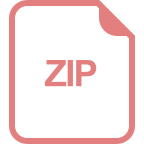
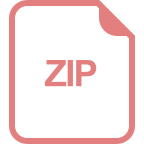
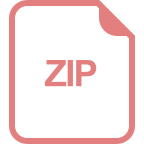
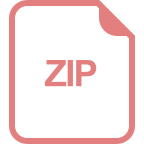
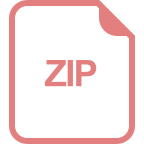
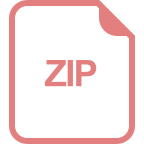