给定一个M乘以N的迷宫图,求从一条指定入口到出口的迷宫路径,在行走中一步只能从当前方块移动到上,下,左,右相邻方块中的一个方快,用栈求一条从指定入口到出口的迷宫路径 C语言
时间: 2024-10-25 07:14:21 浏览: 43
在C语言中,可以使用深度优先搜索(Depth First Search, DFS)结合栈数据结构来解决这个问题。下面是一个基本的步骤:
1. 定义一个函数 `findPath(maze, rows, cols, start, end, path)`,其中`maze`表示迷宫矩阵,`rows`和`cols`是行数和列数,`start`和`end`分别是起点和终点的坐标,`path`是存储路径的栈。
2. 初始化路径栈,将起始点加入路径栈中。
3. 使用循环来处理递归过程:
a. 如果当前节点是终点,打印路径并返回;
b. 将当前位置标记为已访问,避免重复进入;
c. 遍历四个相邻的合法(非墙壁、未访问过)方向,尝试向每个方向移动:
i. 对于每个新位置,如果它不在路径中并且是有效的位置,将其加入栈,并继续搜索下一个邻居;
4. 如果无法到达终点,说明路径不存在,返回空。
5. 为了简洁起见,这里假设迷宫矩阵中0表示可以通行的方格,1表示墙。在实际操作中,需要先预处理这个二维数组。
下面是伪代码形式:
```c
void findPath(int maze[rows][cols], int start[2], int end[2], stack<int> &path) {
// ...其他初始化和辅助函数...
if (maze[end[0]][end[1]] == 0 && !path.empty()) {
printPath(path); // 打印路径
return;
}
path.push(end);
maze[end[0]][end[1]] = 1; // 标记已经访问
for (int direction = 0; direction < 4; direction++) {
int newRow = start[0] + (direction == 0 ? -1 : direction == 1 ? 1 : 0),
newCol = start[1] + (direction == 2 ? -1 : direction == 3 ? 1 : 0);
if (newRow >= 0 && newRow < rows && newCol >= 0 && newCol < cols &&
maze[newRow][newCol] == 0 && path.top() != (newRow << 8 | newCol)) {
dfs(maze, rows, cols, newRow, newCol, path);
}
}
path.pop(); // 回溯检查其他路径
}
```
阅读全文
相关推荐
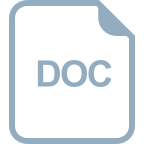
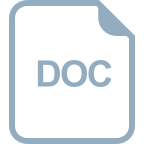
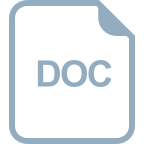
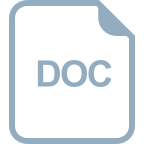
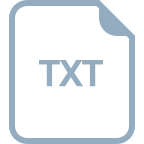
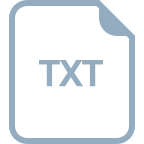
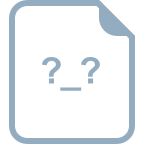
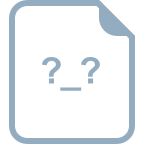
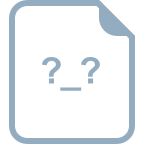
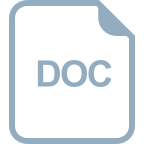
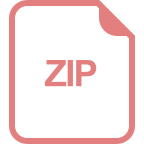
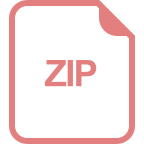
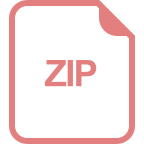
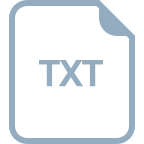
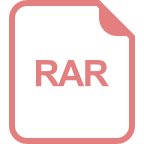
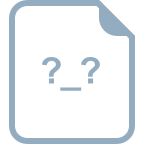
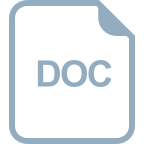