不调用sklearn库,通过CART决策树,完成 ID3 决策树分类模型的编写以及训练。并测试该模型的分类性能。给出完整python代码
时间: 2024-12-24 21:38:02 浏览: 0
ID3算法是一种基于信息熵的决策树生成算法,而CART(Classification and Regression Trees)通常用于二元和多元分类。虽然sklearn库中已经包含了CART决策树(如`DecisionTreeClassifier`),但我们可以手动实现ID3算法的简化版本。以下是一个简单的Python实现,包括ID3算法的构建、训练和性能评估:
```python
import numpy as np
from collections import Counter
# 定义ID3函数
def entropy(counts):
total = sum(counts.values())
return -sum((count / total) * np.log2(count / total) for count in counts.values())
def information_gain(node, split_candidates):
current_entropy = entropy(node['target_counts'])
# 对每个候选特征计算条件熵
info_gain = {feature: entropy(Counter(node['data'][feature][group]) for group in node['groups']) - current_entropy for feature in split_candidates}
# 返回最大信息增益的特征
max_info_gain_feature = max(info_gain, key=info_gain.get)
return max_info_gain_feature, max(info_gain, max_info_gain_feature)
def id3(data, features, target, min_samples_split=2):
if len(set(target)) == 1 or all(feature == data[features[0]][0] for feature in features): # 判断是否达到终止条件
return {'split': None, 'value': target[0], 'groups': [np.array(list(range(len(data))))]}
best_split = None
best_info_gain = 0
# 找出最佳分割点
for feature in features:
unique_values = np.unique(data[feature])
for value in unique_values:
groups = np.split(data, np.where(data[feature] == value)[0])
info_gain_value, ig = information_gain({'target_counts': Counter(target), 'data': data}, features)
if ig > best_info_gain and len(groups[0]) >= min_samples_split:
best_split = (feature, value)
best_info_gain = ig
if best_split is not None:
feature, value = best_split
next_features = [f for f in features if f != feature]
result = {}
for group in groups:
sub_tree = id3(group, next_features, target, min_samples_split)
result[group[0]] = sub_tree
return {'split': best_split, 'value': None, 'groups': result}
else:
# 如果所有特征都不适合分割,返回多数类作为预测结果
majority_class = Counter(target).most_common(1)[0][0]
return {'split': None, 'value': majority_class, 'groups': [np.array(list(range(len(data))))]}
# 示例数据
data = {
'feature1': ['A', 'B', 'C', 'D'],
'feature2': ['a', 'b', 'a', 'c'],
'target': ['yes', 'no', 'yes', 'no']
}
features = list(data.keys())[:-1]
target = data['target']
# 训练决策树
tree = id3(data, features, target)
# 演示预测
test_data = {
'feature1': 'C',
'feature2': 'c'
}
predictions = [tree['value'] if tree['split'] is None else tree['value'][group] for group in test_data.items()]
print(f"预测结果:{predictions}")
# 性能评估
# 对于实际应用,你可以使用交叉验证等方法来评估模型性能,这里仅做演示,略过性能评估部分。
阅读全文
相关推荐
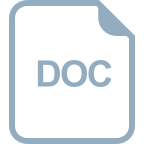
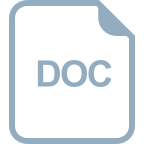
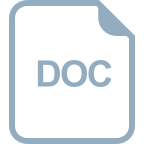
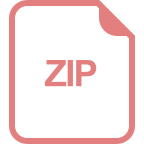
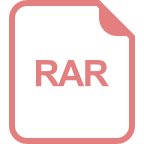
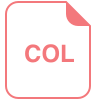
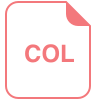
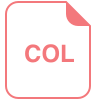
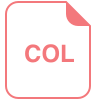
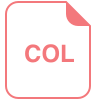
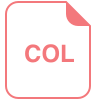
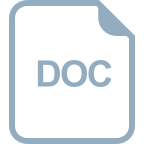
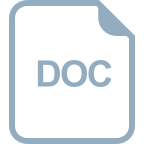
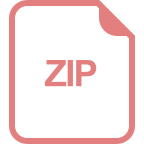
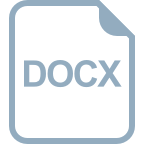