如何使用C语言实现时、分、秒的转换
时间: 2024-10-19 07:12:41 浏览: 34
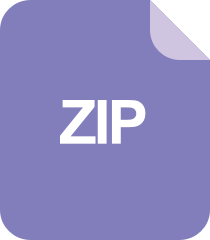
C语言实现秒与时间的互相转换
在C语言中,可以使用结构体和函数来实现时、分、秒之间的转换。首先,定义一个包含时间属性的结构体,例如:
```c
struct Time {
int hours;
int minutes;
int seconds;
};
```
然后,你可以编写两个函数,一个用于将秒转换为时分秒,另一个用于从用户输入获取秒并转换。
**将秒转换为时分秒:**
```c
void convertSecondsToTime(int total_seconds) {
struct Time time;
int remaining_minutes = total_seconds % 60; // 剩余分钟
int remaining_hours = total_seconds / 3600; // 剩余小时
time.hours = remaining_hours;
time.minutes = remaining_minutes % 60;
time.seconds = total_seconds % 60;
// 输出时间
printf("Hours: %d, Minutes: %d, Seconds: %d\n", time.hours, time.minutes, time.seconds);
}
```
**从秒获取用户输入并转换:**
```c
int getUserInput() {
int total_seconds;
printf("Enter the number of seconds: ");
scanf("%d", &total_seconds);
return total_seconds;
}
int main() {
int input_seconds = getUserInput();
convertSecondsToTime(input_seconds);
return 0;
}
```
阅读全文
相关推荐
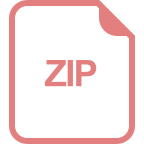
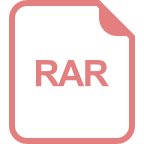
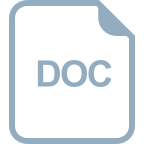
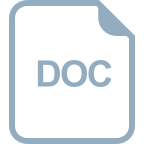
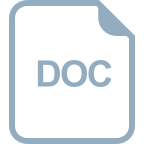
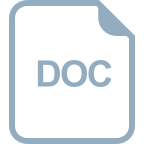
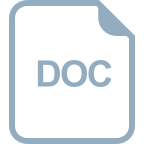
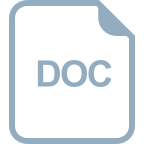
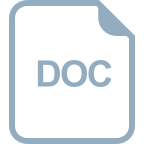








