如何在Android Studio中实现http网络通信?
时间: 2023-11-22 22:48:04 浏览: 30
在Android Studio中实现http网络通信可以通过HttpURLConnection类来实现。具体步骤如下:
1. 在AndroidManifest.xml文件中添加网络权限:
```xml
<uses-permission android:name="android.permission.INTERNET" />
```
2. 在子线程中使用HttpURLConnection类来发送http请求并获取响应:
```java
try {
URL url = new URL("http://www.example.com");
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET"); // 设置请求方法为GET
conn.setConnectTimeout(5000); // 设置连接超时时间为5秒
conn.setReadTimeout(5000); // 设置读取超时时间为5秒
conn.connect(); // 开始连接
int responseCode = conn.getResponseCode(); // 获取响应码
if (responseCode == HttpURLConnection.HTTP_OK) { // 如果响应码为200
InputStream inputStream = conn.getInputStream(); // 获取输入流
BufferedReader reader = new BufferedReader(new InputStreamReader(inputStream));
StringBuilder response = new StringBuilder(); String line;
while ((line = reader.readLine()) != null) {
response.append(line);
}
reader.close();
inputStream.close();
String result = response.toString(); // 将响应结果转换为字符串
// 处理响应结果
} else {
// 处理错误情况
}
} catch (Exception e) {
e.printStackTrace();
}
```
以上代码中,我们首先创建一个URL对象,然后使用openConnection()方法获取HttpURLConnection对象,设置请求方法、连接超时时间和读取超时时间,最后调用connect()方法开始连接。如果响应码为200,说明请求成功,我们可以通过getInputStream()方法获取输入流,然后使用BufferedReader类来读取响应结果并转换为字符串。如果响应码不为200,说明请求失败,我们需要处理错误情况。
相关推荐
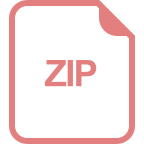
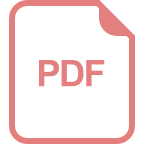














