idea编写java类购物结算系统
时间: 2024-01-13 22:18:31 浏览: 95
以下是使用IntelliJ IDEA编写Java类购物结算系统的步骤:
1.创建一个Java项目并添加所需的依赖项,例如mybatis和mysql驱动程序。
2.创建一个名为“Shopping”的包,并在其中创建以下类:
- 商品类(Product):包含商品的名称,价格和数量等属性。
- 购物车类(ShoppingCart):包含添加商品,删除商品和计算总价等方法。
- 主类(Main):包含主方法,用于测试购物车类。
3.在mybatis中创建一个名为“product”的表,用于存储商品信息。
4.创建一个名为“ProductMapper”的接口,并在其中定义用于从数据库中检索商品信息的方法。
5.创建一个名为“ProductMapper.xml”的文件,并在其中定义与ProductMapper接口中方法相对应的SQL语句。
6.在ShoppingCart类中,使用mybatis和ProductMapper接口从数据库中检索商品信息,并将其添加到购物车中。
7.在Main类中,创建一个ShoppingCart对象,并向其中添加一些商品。然后,调用计算总价的方法,并将结果打印到控制台上。
```java
// Product.java
public class Product {
private String name;
private double price;
private int quantity;
// 构造函数
public Product(String name, double price, int quantity) {
this.name = name;
this.price = price;
this.quantity = quantity;
}
// getter和setter方法
// ...
// 计算商品总价
public double getTotalPrice() {
return price * quantity;
}
}
// ShoppingCart.java
public class ShoppingCart {
private List<Product> productList = new ArrayList<>();
// 添加商品
public void addProduct(Product product) {
productList.add(product);
}
// 删除商品
public void removeProduct(Product product) {
productList.remove(product);
}
// 计算总价
public double getTotalPrice() {
double totalPrice = 0;
for (Product product : productList) {
totalPrice += product.getTotalPrice();
}
return totalPrice;
}
}
// Main.java
public class Main {
public static void main(String[] args) {
// 创建购物车对象
ShoppingCart cart = new ShoppingCart();
// 从数据库中检索商品信息,并添加到购物车中
SqlSession sqlSession = MybatisUtil.getSqlSession();
ProductMapper productMapper = sqlSession.getMapper(ProductMapper.class);
List<Product> productList = productMapper.getProductList();
for (Product product : productList) {
cart.addProduct(product);
}
// 计算总价并打印结果
double totalPrice = cart.getTotalPrice();
System.out.println("Total price: " + totalPrice);
}
}
```
阅读全文
相关推荐



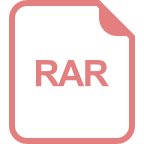


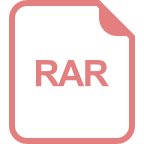












