请利用Python编写一个可以实现 图像识别功能的app
时间: 2024-12-20 07:23:05 浏览: 3
在Python中实现图像识别通常涉及到机器学习和深度学习技术,尤其是卷积神经网络(CNN)。以下是一个简单示例,展示如何使用预训练模型如TensorFlow的`tf.keras`库进行图像分类。假设我们想识别猫狗图片,可以采用经典的Keras `MobileNetV2` 模型。
```python
# 首先,确保已安装必要的库
!pip install tensorflow numpy pillow
import tensorflow as tf
from tensorflow.keras.applications.mobilenet_v2 import MobileNetV2, preprocess_input
from tensorflow.keras.preprocessing.image import ImageDataGenerator
from tensorflow.keras.models import Model
from tensorflow.keras.layers import Dense, GlobalAveragePooling2D
import numpy as np
import os
# 1. 加载预训练模型
base_model = MobileNetV2(weights='imagenet', include_top=False, input_shape=(224, 224, 3))
# 2. 添加新的全连接层用于特定任务
x = base_model.output
x = GlobalAveragePooling2D()(x)
predictions = Dense(2, activation='softmax')(x) # 这里2代表猫和狗两个类别
# 创建新模型
model = Model(inputs=base_model.input, outputs=predictions)
# 冻结预训练模型的权重
for layer in base_model.layers:
layer.trainable = False
# 编译模型
model.compile(optimizer=tf.keras.optimizers.Adam(), loss='categorical_crossentropy', metrics=['accuracy'])
# 准备数据集
train_dir = 'path_to_train_images'
validation_dir = 'path_to_validation_images'
train_datagen = ImageDataGenerator(preprocessing_function=preprocess_input)
val_datagen = ImageDataGenerator(preprocess_function=preprocess_input)
train_generator = train_datagen.flow_from_directory(
train_dir,
target_size=(224, 224),
batch_size=32,
class_mode='categorical')
validation_generator = val_datagen.flow_from_directory(
validation_dir,
target_size=(224, 224),
batch_size=32,
class_mode='categorical')
# 训练模型
history = model.fit(
train_generator,
epochs=10, # 调整epochs根据实际情况
validation_data=validation_generator)
# 3. 实际应用:预测新图片
def predict_image(image_path):
img = tf.keras.preprocessing.image.load_img(image_path, target_size=(224, 224))
x = tf.keras.preprocessing.image.img_to_array(img)
x = np.expand_dims(x, axis=0)
x = preprocess_input(x)
predictions = model.predict(x)
predicted_class = np.argmax(predictions)
print(f"Predicted class: {predicted_class}")
# 使用predict_image函数识别新图片
predict_image('path_to_test_image.jpg')
阅读全文
相关推荐
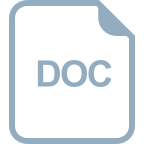
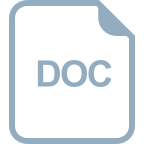
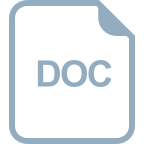

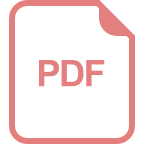
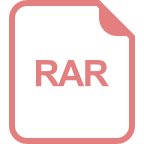
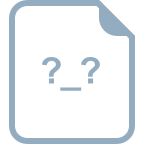
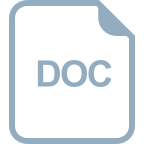
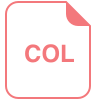
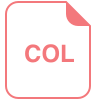
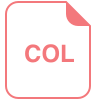
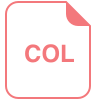






