c++ map下标遍历
时间: 2023-11-01 19:56:04 浏览: 124
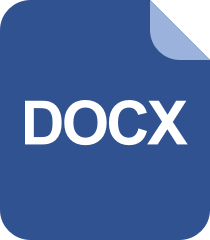
C++ map使用

遍历C++的map容器可以使用迭代器来实现。下面是一个关于如何通过迭代器遍历map容器的示例代码:
```cpp
#include <map>
#include <iostream>
int main() {
std::map<int, std::string> mapStudent;
mapStudent = "student_one";
mapStudent = "student_two";
mapStudent = "student_three";
std::map<int, std::string>::iterator iter;
for(iter = mapStudent.begin(); iter != mapStudent.end(); ++iter) {
std::cout << iter->first << " " << iter->second << std::endl;
}
return 0;
}
```
上述代码通过迭代器`iter`来遍历map容器`mapStudent`,并输出每个键值对的值。在循环中,`iter->first`表示当前迭代器指向的键,`iter->second`表示当前迭代器指向的值。通过输出这两个值,即可遍历整个map容器。
阅读全文
相关推荐
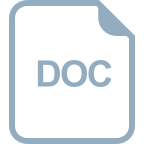
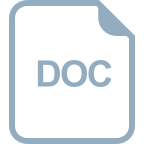



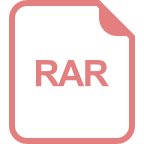
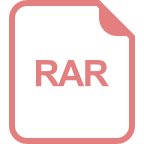
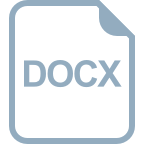
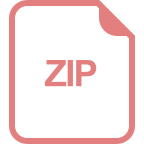
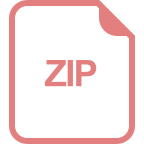
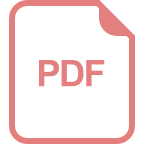






