头歌循环队列及链队列的基本操作答案你有没有
时间: 2024-09-28 20:16:11 浏览: 71
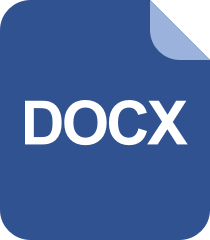
头歌数据结构循环队列及链队列的基本操作

头歌课程中确实涵盖了链队列和循环队列的基本操作。对于链队列,关键函数可能包括:
1. 初始化:创建一个新的空链队列[^1]。
```python
class Queue:
def __init__(self):
self.head = None
self.tail = None
```
2. **入队(EnQueue)**:将元素添加到队尾。
```python
def enqueue(self, item):
new_node = Node(item)
if not self.is_full():
if self.isEmpty():
self.head = new_node
self.tail = new_node
else:
self.tail.next = new_node
self.tail = new_node
```
3. **出队(DeQueue)**:从队头移除并返回元素。
```python
def dequeue(self):
if self.isEmpty():
return None
removed_item = self.head.data
if self.head == self.tail:
self.head = None
self.tail = None
else:
self.head = self.head.next
return removed_item
```
4. **判断队列是否为空(QueueEmpty)**:
```python
def isEmpty(self):
return self.head is None
```
5. **获取队列元素个数(QueueLength)**:
```python
def size(self):
count = 0
current = self.head
while current:
count += 1
current = current.next
return count
```
6. **检查队列是否已满(is_full)**:
```python
def is_full(self):
# 假设队列的容量为capacity
if (self.tail - self.head + 1) >= capacity:
return True
else:
return False
```
7. **遍历队列(QueueTraverse)**:这通常是通过迭代节点来完成的,但这里没有直接给出代码,你可以自己实现一个for循环遍历。
以上是一些基本操作的示例,实际实现可能需要具体的数据结构定义(如Node类),以及对队列边界条件的处理。
阅读全文
相关推荐
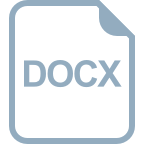
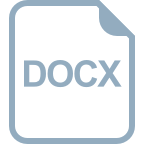


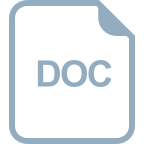
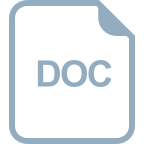



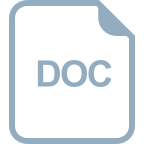
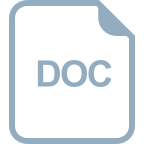
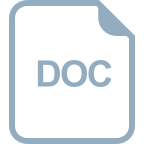




